When it comes to dynamic programming languages like Python, one of the key concepts that developers often encounter is “Duck Typing.” Duck typing is a flexible and powerful approach to variable type checking that can simplify your code and make it more robust.
Understanding the principles of Duck Typing in Python is essential as it forms the basis for grasping the concept of Python Singletons in the subsequent article. In this article, we will explore the ins and outs of duck typing and how it can be used effectively to check variable types in Python.
Duck Typing in Python: Flexibility and Power in Dynamic Typing
When it comes to dynamic programming languages like Python, one of the key concepts that developers often encounter is “Duck Typing.” Duck typing is a flexible and powerful approach to variable type checking that can simplify your code and make it more robust. In this article, we will explore the ins and outs of duck typing and how it can be used effectively to check variable types in Python.
The name duck typing has its root in the expression If it walks like a duck and it quacks like a duck, then it must be a duck. Which means that if you want to know whether you are dealing with a duck or not, you only care about how it looks like and how it behaves rather than actually checking to what species the animal belongs.
The analogy holds true in Python, which is a dynamically typed language, where you don’t need to specify variable types explicitly. In Python, you can seamlessly change the type of a variable as needed, as demonstrated in the following code:
var1 = 'This is a string'
var1 = 1
In the example above, var1 transitions effortlessly from a string to an integer without requiring any explicit type declarations. This dynamic typing feature is convenient, as it reduces the need for verbose type annotations. However, it comes with a trade-off: Python doesn’t provide compile-time type checking, which means we may not be aware of variable type issues until runtime.
For instance, consider this function:
def increase_by_one(value)
return value + 1
When we invoke increase_by_one with var1, it will execute successfully only if var1 is a numeric type (either an integer or a float). If var1 contains a string, it will raise a TypeError. In contrast, statically typed languages would catch such type inconsistencies during compilation, potentially preventing future debugging challenges.
To address this issue in Python, one approach is to explicitly check whether the value is an integer or a float within the function, like this:
def increase_by_one(value):
if isinstance(value, int) or isinstance(value, float):
return value + 1
With this modification, if you attempt to run the function with a string input, it will gracefully handle the situation by returning None. For numeric inputs (integers or floats), it will increase the value by one as expected. This behavior aligns more closely with our intended functionality, enhancing the robustness of our code.
What is Duck Typing?
After this preliminary discussion, you might be eager to delve into the world of duck typing and truly comprehend what it entails. So, what exactly is duck typing? Let’s embark on a journey to demystify this concept.
Imagine, for a moment, that we possess a NumPy array, and we decide to employ the increase_by_one function with it. What do you anticipate will occur?
import numpy as np
var1 = np.array((0, 1, 2))
print(increase_by_one(var1))
Surprisingly, you receive a None output. But is this the outcome you were expecting? It’s worth reflecting on the fact that we have deliberately shaped the function’s behavior to function only with integers and floats, while a NumPy array fits neither of these categories. However, if we revert to the earlier version of the function, the one before we introduced this explicit verification, something fascinating unfolds:
def increase_by_one(value):
return value + 1
print(increase_by_one(var1))
# [1, 2, 3]
Astoundingly, the increase_by_one function performs seamlessly with arrays as well. Duck typing, in essence, signifies that we need not concern ourselves with the specific type of variable value is, as long as we can add 1 to it. In Python, this philosophy translates to attempting to add 1 to value and, if an exception arises, handling it gracefully:
def increase_by_one(value):
try:
value += 1
except TypeError:
return None
return value
With this approach, the function accommodates an array of variable types that can gracefully accept the addition of one. Initially, we may have presumed that only floats and integers possessed this capability, but as we’ve seen, NumPy arrays and various other data structures can also participate in this duck typing dance. The beauty lies in the flexibility and adaptability that duck typing brings to our code, allowing us to focus on the operations we can perform rather than being bogged down by strict type constraints. The world of duck typing is indeed a realm of endless possibilities.
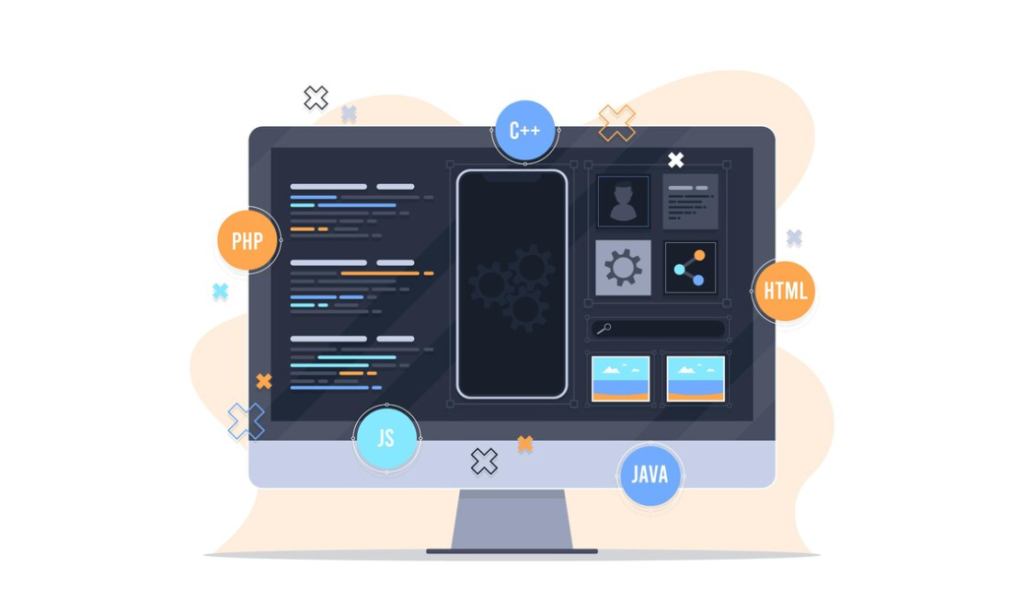
Duck Typing in Custom Classes: Crafting Dynamic Behaviors
Duck typing takes on a pivotal role when you venture into the realm of developing custom classes in Python. This language offers a wealth of syntactic sugar that empowers you to customize how objects behave during various operations. To illustrate the concept further, let’s embark on a journey to create a class that can be incremented by one:
class AddOne:
def __init__(self, value):
self.value = str(value)
def __add__(self, other):
self.value += str(other)
return self
def __str__(self):
return self.value
Now, let's put our custom class to the test:
var1 = AddOne('0')
print(increase_by_one(var1))
# 01
What you observe here is the class’s ability to define the behavior of addition. In this instance, our class concatenates any value we add to the initial string we’ve defined. This is precisely why we witness the output “01.”
The essence of duck typing shines through in the fact that our increase_by_one function operates every time there’s a viable way to add 1 to the object. While the example in these sections may appear relatively simple, it paves the way for a deeper exploration of a concept that becomes immensely relevant as you dive further into the world of custom classes and dynamic behaviors. The possibilities are virtually boundless, and duck typing remains a powerful tool in crafting adaptable and versatile Python code.
Complex Data Structures: Managing Configuration Variables
As you embark on the journey of developing larger and more intricate software programs, the need for configuration variables becomes almost inevitable. These variables play a vital role in tailoring your program’s behavior to specific requirements and scenarios. Storing these crucial configuration parameters in dictionaries proves to be an elegant and explicit solution, providing a streamlined way to manage your program’s settings.
Let’s break it down to its simplest form:
configuration = {
'param1': 10,
'param2': 5
}
Additionally, an Experiment class is crafted to utilize the configuration and conduct essential checks to verify the presence of all necessary parameters.
class Experiment:
def __init__(self, config):
self.configuration = config
def check_config(self):
if not {'param1', 'param2'} <= set(self.configuration.keys()):
raise Exception('The configuration does not include the mandatory fields')
print('Config seems OK')
Notice the implementation of the check_config function, which employs sets to ascertain the presence of both parameters within the dictionary’s keys. In essence, it validates if the set {‘param1’, ‘param2’} is a subset of all the keys within the configuration.
To enhance the utility of our class, we can introduce a final method for validating whether the parameters fall within an acceptable range:
def check_config_range(self):
if self.configuration['param1'] > 10:
raise Exception('param1 cannot be larger than 10')
if self.configuration['param2'] > 5:
raise Exception('param2 cannot be larger than 5')
print('Range seems OK')
You can evaluate the code’s functionality by executing the following:
exp = Experiment(configuration)
exp.check_config()
exp.check_config_range()
So, how does duck typing come into play here? In the aforementioned code, an assumption is made that the configuration will be a dictionary. However, we are not confined to this assumption. Picture a scenario where we aspire to enhance our configuration handling capabilities. We might want to create a custom class responsible for reading from a file, logging parameter changes, and more. The objective is to ensure compatibility with the existing Experiment class we’ve developed. In this context, duck typing operates in a reverse manner. We understand what the Experiment class requires to function effectively, and we design a solution tailored to those requirements.
If you examine the Experiment code closely, you’ll notice it interacts with the configuration in two distinct instances. Firstly, it checks for the presence of both param1 and param2 through the keys method. We already know that we need a class that supports this method:
class Config:
def __init__(self):
...
def keys(self):
...
Furthermore, it’s worth noting that when accessing parameters, they are retrieved via configuration[‘param1’]. To enable this behavior, adjustments to the magic method __getitem__ are necessary. Now, let’s introduce an additional requirement: we desire the ability to instantiate this class with a filename. This filename will be processed by the class, loading its data. For the sake of simplicity, we enforce that the configuration file must adhere to the YAML format. As a result, our Config class takes on the following form (please ensure you have pyyaml installed for this functionality to operate correctly):
If we break down the process step by step, it becomes evident that upon instantiating the class, we request a filename. This filename is subsequently opened, and its contents are loaded into an attribute known as _config. It’s important to note that Python does not have true private attributes for classes, i.e., attributes that can only be accessed within the class and not from outside. As a convention, attributes starting with an underscore, such as _config, indicate that they are not intended for direct external use, although this convention cannot be strictly enforced.
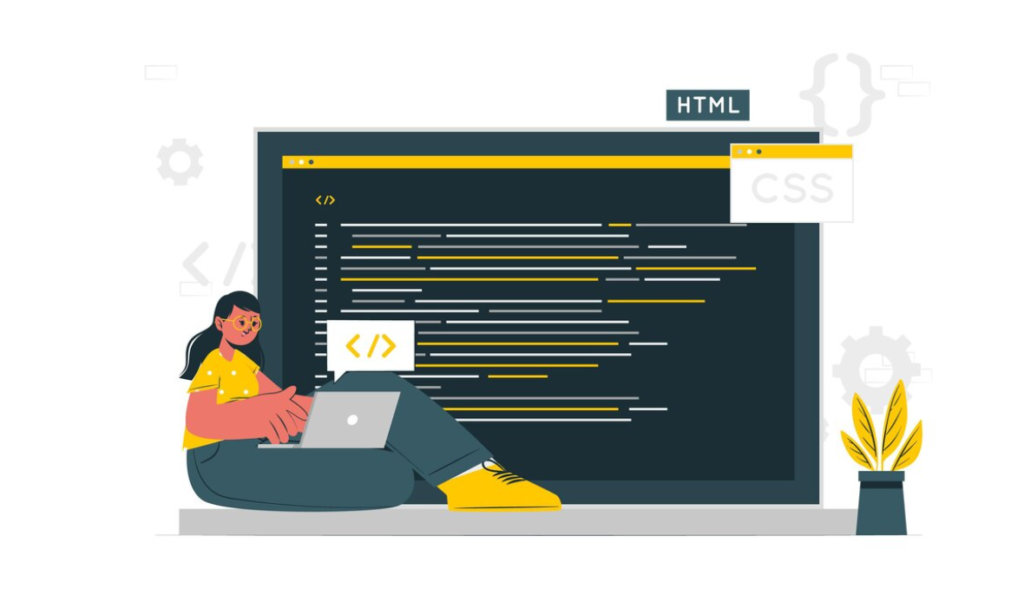
Given that _config is a dictionary, implementing the keys method is straightforward as we can utilize the default dictionary method. However, __getitem__ is considerably more intriguing. In Python, the __getitem__ method governs what occurs when you attempt something like c[‘param1’]. In this case, the item corresponds to param1, and our objective is to retrieve that specific item from the _config dictionary. To assess this implementation, you must first create a file named config.yml with the following contents:
param1: 10
param2: 5
Afterward, you can execute the following commands:
c = Config('config.yml')
print(c['param1'])
print(c['[param2'])
However, it’s important to note that attempting to modify the values of ‘param1’ or ‘param2’ will result in an error. Delving into this topic goes beyond the scope of duck typing, so stay tuned as we plan to address it in a future tutorial.
Now, let’s integrate all the components we’ve discussed – our custom configuration class and the experiment class:
c = Config('config.yml')
exp = Experiment(c)
exp.check_config()
exp.check_config_range()
You can observe that the Experiment is now operating with a configuration that isn’t a dictionary but a custom-designed class, and it functions as expected.
Conclusions
When delving into the realm of duck typing, a prevalent principle in Python, the conventional wisdom often holds that one should not be overly concerned with ascertaining the precise data type of a variable. Instead, the emphasis lies on ensuring that the variables exhibit the expected behaviors. This approach simplifies code implementation significantly. As demonstrated earlier, it enables the utilization of functions on variables that might not have been initially intended recipients, including data structures like NumPy arrays and custom classes. The flexibility and adaptability that duck typing provides are invaluable assets for Python programmers, fostering a dynamic and efficient coding environment.