When working on larger programming projects, understanding certain programming patterns can be crucial for preemptively solving potential issues. A key pattern in this context is the concept of singletons. Singletons are unique objects in a program that are created only once. Python, interestingly, introduces us to singleton patterns from the very start, often without us realizing it. For developers venturing beyond foundational concepts like the Python Singleton pattern, an exploration into practical applications such as creating graphical user interfaces with OpenCV offers an exciting expansion of skills and tools.
This article will delve into how singletons are an integral part of our daily programming in Python and explore ways to utilize them more effectively.
Understanding Singletons in Daily Use
To grasp singletons effectively, it’s crucial to first understand Python’s approach to mutable and immutable data types. Consider a list in Python – it’s a mutable data type, allowing us to alter its contents without needing to create an entirely new object. For instance:
>>> var1 = [1, 2, 3]
>>> var2 = var1
>>> var1[0] = 0
>>> print(var2)
[0, 2, 3]
If we possess two lists, such as var1 and var2, we can determine if they share identical content.
>>> var1 == var2
True
However, we can also ascertain whether they refer to the same object.
>>> var1 is var2
True
Nevertheless, we also have the option to:
>>> var1 = [1, 2, 3]
>>> var2 = [1, 2, 3]
>>> var1 == var2
True
>>> var1 is var2
False
In this scenario, both var1 and var2 contain identical values [1, 2, 3], yet they represent distinct objects. This is why the expression var1 is var2 returns False.
However, Python developers are typically introduced to the following syntax at an early stage:
if var is None:
print('Var is none')
At first glance, one might wonder why we can use is in the above example. The answer lies in the fact that None is a unique type of object, which can be instantiated only once. Let’s explore some examples:
>>> var1 = None
>>> var2 = None
>>> var1 == var2
True
>>> var1 is var2
True
>>> var3 = var2
>>> var3 is var1
True
>>> var3 is None
True
This implies that within our code, there can exist only one instance of None, and any variable referencing it will point to the same object. This is in contrast to the situation when we created two lists with the same values. Alongside None, the other two common singletons are True and False:
>>> [1, 2, 3] is [1, 2, 3]
False
>>> None is None
True
>>> False is False
True
>>> True is true
True
This wraps up the trio of singletons commonly encountered by Python developers: None, True, and False. This also sheds light on why the ‘is’ operator is used for comparisons with these singletons. However, these examples are just the tip of the iceberg in terms of singleton usage in Python.
Singletons in Small Integers
Python also defines less apparent singletons, primarily for memory and speed optimization. An example is the range of small integers from -5 to 256. This allows for operations like the following:
>>> var1 = 1
>>> var2 = 1
>>> var1 is var2
True
Or, perhaps more intriguingly:
>>> var1 = [1, 2, 3]
>>> var2 = [1, 2, 3]
>>> var1 is var2
False
>>> for i, j in zip(var1, var2):
... i is j
...
True
True
True
In the above example, you observe two lists with identical elements. They are distinct lists from the previous instance, but each element is the same. If we wish to delve into more sophisticated Python syntax (simply because we have the capability), we can also execute the following:
>>> var1 = [i for i in range(250, 260)]
>>> var2 = [i for i in range(250, 260)]
>>> for i, j in zip(var1, var2):
... print(i, i is j)
...
250 True
251 True
252 True
253 True
254 True
255 True
256 True
257 False
258 False
259 False
The behavior of Python’s singletons is intriguing: integers up to 256 share the same identity, but starting from 257, they do not.
Singletons in Short Strings
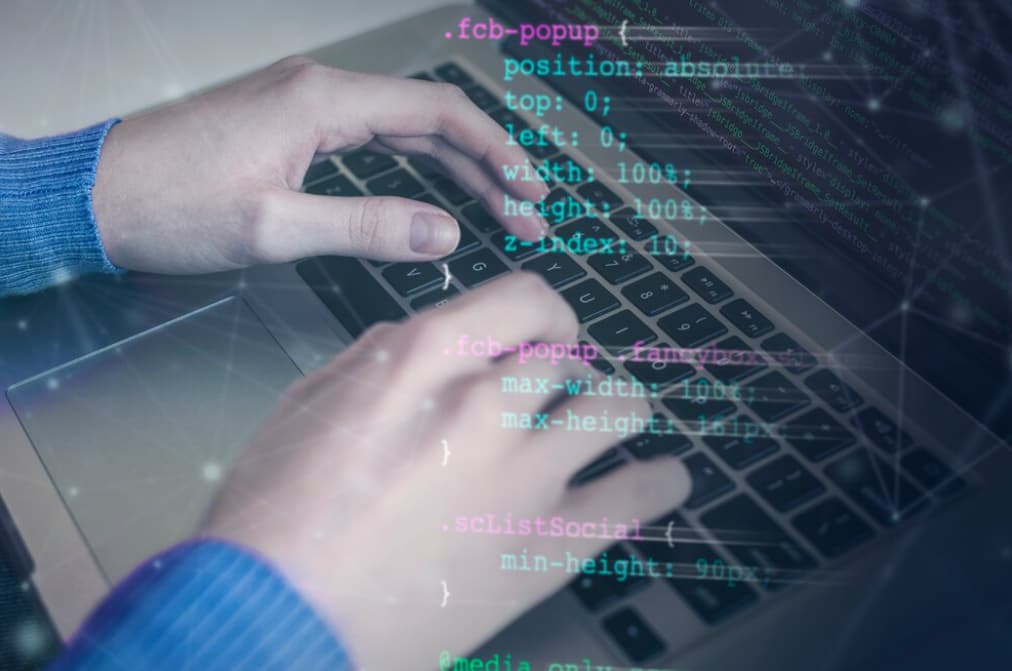
Interestingly, small integers aren’t the only singletons in Python. Short strings can also exhibit singleton properties under certain circumstances. To understand this, consider the following example:
>>> var1 = 'abc'
>>> var2 = 'abc'
>>> var1 is var2
True
The concept of singletons in Python extends to strings, but with a different mechanism known as string interning, detailed on Wikipedia. Python’s approach to allocating memory for strings as singletons is guided by specific rules. Primarily, the strings need to be defined at compile-time, meaning they shouldn’t be generated by formatting operations or functions. For instance, in the example ‘var1 = ‘abc”, the string ‘abc’ is a candidate for interning.
Python’s efficiency extends to interning other strings it deems beneficial for memory (and/or time) savings. A common example of this is the interning of function names:
>>> def test_func():
... print('test func')
...
>>> var1 = 'test_func'
>>> test_func.__name__ is var1
True
By default, empty strings and certain single-character strings are interned, much like small integers.
>>> var1 = chr(255)
>>> var2 = chr(255)
>>> var3 = chr(256)
>>> var4 = chr(256)
>>> var1 is var2
True
>>> var3 is var4
False
Although certain strings are interned, it doesn’t warrant excessive confidence. For instance:
>>> var1 = 'Test String'
>>> var2 = 'Test String'
>>> var1 is var2
False
>>> var2 = 'TestString'
>>> var1 = 'TestString'
>>> var1 is var2
True
As evident in the above example, being a short string is not the sole criterion. The string must also consist of a restricted set of characters, excluding spaces.
Consequently, the interned nature of strings in Python doesn’t imply that we should prefer the is syntax over ==. It simply signifies that Python incorporates certain optimizations behind the scenes. While these optimizations may become relevant for our code one day, they are more likely to go unnoticed but appreciated.
The Purpose and Use of Singletons in Programming
Our exploration so far has highlighted the intriguing aspect of singletons, but it’s essential to understand why they are employed in programming. A primary reason for using singletons is memory efficiency. In Python, variables are more like labels pointing to underlying data. If multiple labels point to the same data, it conserves memory since there’s no duplication of information.
However, the practicality of incorporating a singleton in our code is not always clear. A singleton is a class designed to be instantiated just once. Subsequent instances reference the initial one, making them identical. It’s easy to confuse singletons with global variables, but they differ significantly. Global variables don’t inherently dictate instantiation methods; a global variable could reference one class instance, while a local variable might reference another.
Singletons are a design pattern in programming, offering utility but not indispensability. They can’t accomplish anything that can’t be achieved by other means. A classic example of singleton usage is a logger. Different parts of a program can interact with a single logger instance. This logger then determines whether to output to the terminal, save to a file, or perform no action at all. This is where singletons shine, enabling centralized management and consistent behavior across an application.
Singleton Design Pattern: Ensuring Single Instantiation
The fundamental aspect of singletons lies in the prevention of multiple instantiations. Let’s begin by exploring the consequences of instantiating a class twice:
class MySingleton:
pass
ms1 = MySingleton()
ms2 = MySingleton()
print(ms1 is ms2)
# False
As observed, each instance created in the usual manner is a separate object. To restrict this to a single instantiation, it’s necessary to monitor if the class has already been instantiated. This can be achieved by utilizing a class variable to track the instantiation status and ensure the same object is returned for subsequent requests. One effective approach is to implement this logic in the class’s __new__ method:
class MySingleton:
instance = None
def __new__(cls, *args, **kwargs):
if not isinstance(cls.instance, cls):
cls.instance = object.__new__(cls)
return cls.instance
And we can verify this:
>>> ms1 = MySingleton()
>>> ms2 = MySingleton()
>>> ms1 is ms2
True
This method for implementing a singleton is quite direct. The key step involves checking if an instance already exists; if not, it’s created. While it’s possible to use other variables like __instance or more complex checks to determine the instance’s existence, the outcome remains consistent.
However, it’s important to note that the practicality of singletons as a design pattern can often be challenging to justify. To illustrate, consider a scenario where a file needs to be opened multiple times. In such a case, a singleton class would be structured as follows:
class MyFile:
_instance = None
file = None
def __init__(self, filename):
if self.file is None:
self.file = open(filename, 'w')
def write(self, line):
self.file.write(line + '\n')
def __new__(cls, *args, **kwargs):
if not isinstance(cls._instance, cls):
cls._instance = object.__new__(cls)
return cls._instance
It’s important to highlight a few aspects of this implementation.
- Firstly, the ‘file’ is defined as a class attribute, not an instance attribute;
- This distinction is crucial because the __init__ method gets executed each time the class is instantiated;
- By setting ‘file’ as a class attribute, we ensure the file is opened only once;
- This behavior can also be replicated directly in the __new__ method, after verifying the _instance attribute;
- Additionally, note that the file is opened in ‘w’ mode, signifying that its contents will be overwritten each time.
The singleton can be employed as follows:
>>> f = MyFile('test.txt')
>>> f.write('test1')
>>> f.write('test2')
>>> f2 = MyFile('test.txt')
>>> f2.write('test3')
>>> f2.write('test4')
The above example demonstrates that the order of defining ‘f’ or ‘f2’ is irrelevant in the context of our singleton pattern. The key point is that the file is opened just once. As a result, its contents are cleared a single time, and subsequent writes through the program append lines to the file. After executing the given code, the file content will be:
test1
test2
test3
test4
This consistently appended output confirms the singleton behavior. Additionally, we can verify the singleton nature of our implementation as follows:
>>> f is f2
True
Nevertheless, in the manner we outlined our class earlier, a significant issue arises. What would be the output of the following?
>>> f = MyFile('test.txt')
>>> f.write('test1')
>>> f.write('test2')
>>> f2 = MyFile('test2.txt')
>>> f2.write('test3')
>>> f2.write('test4')
The provided code functions correctly, but it’s important to note that the program will create only ‘test.txt’ due to the singleton pattern and effectively disregard the argument provided for the second instantiation. This is a direct result of the singleton’s nature, where only the first instantiation’s parameters are considered, and subsequent attempts use the same instance.
An intriguing consideration arises when pondering the removal of the __new__ method from the implementation. Let’s explore what the implications of this change would be:
class MyFile:
file = []
def __init__(self, filename):
if len(self.file) == 0:
self.file.append(open(filename, 'w'))
def write(self, line):
self.file[0].write(line + '\n')
By definition, this class is not a singleton, as each instantiation results in a different object:
>>> f = MyFile('test.txt')
>>> f2 = MyFile('test.txt')
>>> f is f2
False
>>> f.write('test1')
>>> f.write('test2')
>>> f2.write('test3')
>>> f2.write('test4')
This approach subtly shifts the strategy by changing the file attribute from ‘None’ to an empty list, leveraging the mutable nature of lists. When the opened file is appended to this list, the list remains the same object, thus shared across all instances. Despite this modification, the overall outcome remains unchanged: the file is opened only once, and lines are appended as before.
The key takeaway from this example is that the functionality of opening a file just once isn’t exclusive to singletons. By intelligently utilizing the concept of mutability, the same effect can be achieved with even less code.
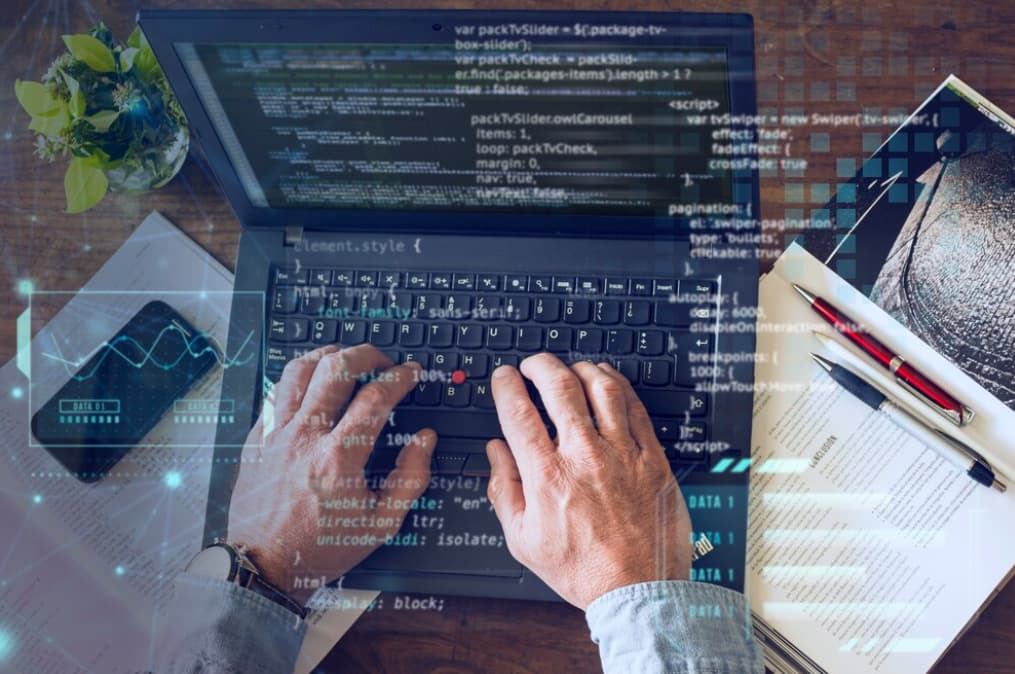
Singletons in Python: Efficiency in Lower-Level Applications
The singleton pattern plays a significant role in the development of lower-level applications and frameworks. Python itself employs singletons to enhance execution speed and improve memory efficiency. A notable observation is the time taken to evaluate expressions like f == f2 versus f is f2 in singleton versus non-singleton scenarios. Typically, there’s a noticeable time benefit in the former case. The impact of these optimizations on the overall costs and limitations largely depends on the frequency of equality checks within the application.
In contrast, finding applications of the singleton pattern in higher-level programming can be more challenging. The most commonly cited example is the implementation of loggers. Beyond this, examples in higher-level projects are not as prevalent. It would indeed be insightful to learn about other instances where singletons have been effectively used in high-level programming contexts.
Singletons and Their Impact on Unit Testing
It’s important to note that the singleton pattern can inadvertently disrupt the integrity of unit tests. Consider the singleton example previously discussed. If one were to modify the MyFile object, say by executing f.new_file = open(‘another_file’), this alteration would be persistent and could influence subsequent tests. The fundamental principle of unit testing is that each test should be isolated, focusing solely on one aspect. When tests have the potential to affect each other, they no longer adhere to the strict definition of unit tests, thereby compromising their reliability and effectiveness.
Conclusion
Singletons provide an interesting way of creating objects that only exist once in Python. They’re a powerful tool used for memory and speed efficiency. However, their usage needs to be thought through carefully due to potential pitfalls. Understanding when and how to use singletons can greatly aid in creating more efficient and robust Python code.