Introduction
Python, known for its versatility and ease of use, sometimes faces criticism for not being the fastest language around. However, there’s a feature in Python that’s often overlooked but can significantly improve performance: using slots. This article dives deep into how using slots in Python can limit dynamic attribute creation and enhance speed, offering a practical approach to optimizing your Python code.
Understanding the Basics of Slots in Python
Before diving into the intricacies of slots in Python, it’s essential to grasp the fundamentals. Python is known for its dynamic nature, where objects can have attributes added to them on the fly. While this flexibility is advantageous, it also comes with a trade-off in terms of memory and speed. This is where the concept of slots becomes significant.
What are Slots?
Slots in Python provide a mechanism for restricting objects to a fixed set of attributes. When you define a class with slots, you are explicitly specifying the attributes that instances of that class can possess, and nothing more. This constraint leads to more efficient memory usage and faster attribute access.
Key Advantages of Using Slots:
- Memory Efficiency: If slots aren’t available, class instances can dynamically store their characteristics in a dictionary-like fashion using the __dict__ attribute. The memory efficiency can be negatively affected by this dynamic nature, particularly when working with a high number of instances. By substituting a statically specified list of properties for the __dict__, slots significantly reduce memory use;
- Faster Attribute Access: The absence of a dynamic dictionary improves attribute access speed. With slots, attribute access is direct and doesn’t involve dictionary lookups, making it significantly faster.
How Slots Work
To comprehend how slots work in Python, it’s crucial to understand the conventional way of defining classes and how slots provide an alternative approach.
In a standard class definition in Python, each instance of the class has a __dict__ attribute by default. This __dict__ attribute acts as a dictionary to store all the object’s attributes. Here’s an example:
class Person: def __init__(self, name, age): self.name = name self.age = age p1 = Person(“Alice”, 30) p2 = Person(“Bob”, 25) |
In this case, both p1 and p2 have their own __dict__ dictionaries to store name and age.
Using Slots (Efficient Approach)
When slots are employed, you define a class with a special attribute called __slots__, which is a tuple containing the names of the allowed attributes. Here’s an example:
class PersonWithSlots: __slots__ = (‘name’, ‘age’) def __init__(self, name, age): self.name = name self.age = age p1 = PersonWithSlots(“Alice”, 30) p2 = PersonWithSlots(“Bob”, 25) |
In this case, instances of PersonWithSlots can only have the attributes name and age. The class no longer uses the memory-consuming __dict__ dictionary, resulting in improved memory efficiency and faster attribute access.
When to Use Slots
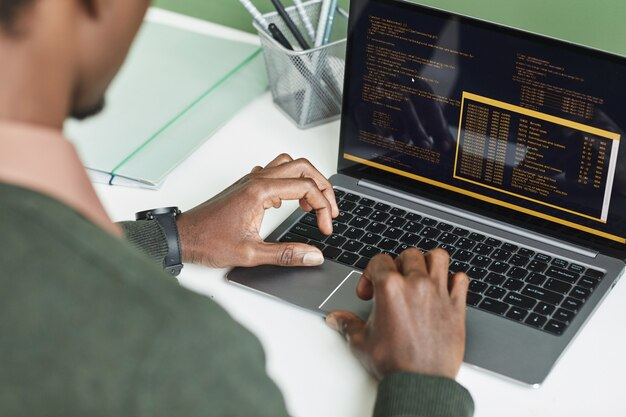
Slots are a valuable tool in Python, but they are not always necessary. You should consider using slots in the following situations:
Memory Efficiency Concerns
Memory consumption can be a critical concern, especially when dealing with a large number of class instances. By default, in Python, each instance stores its attributes in a dictionary, which incurs a memory overhead. Slots address this issue by replacing the dictionary with a more memory-efficient structure.
Without Slots | With Slots |
---|---|
Each instance uses a dictionary to store attributes, consuming extra memory. | Slots define a fixed attribute set, eliminating the need for individual dictionaries. |
Memory overhead increases with the number of instances. | Memory overhead remains constant regardless of the number of instances. |
Ideal for applications with many instances, such as database records or large datasets. | Significantly reduces memory consumption in situations where memory is a concern. |
Performance Optimization
In performance-critical applications, the speed of attribute access can become a bottleneck. Slots offer a solution to this problem by improving attribute access speed. Without slots, Python’s interpreter needs to perform dictionary lookups to access attributes. Slots eliminate these lookups by allowing direct attribute access with a fixed memory layout.
Without Slots | With Slots |
---|---|
Attribute access involves dictionary lookups, which can be slower. | Direct attribute access without dictionary lookups, resulting in faster access. |
Slower attribute access can impact the overall performance. | Ideal for performance-critical applications, ensuring efficient attribute access. |
Particularly useful when frequently accessing attributes in loops. | Helps optimize code execution and reduce bottlenecks in attribute access. |
Restricting Attributes
Slots are also valuable when you want to enforce a strict set of attributes for instances and prevent the addition of arbitrary attributes. This can be crucial for maintaining data integrity and preventing accidental modifications to your class instances. With slots, you explicitly define the allowed attributes, and any attempt to add new attributes will result in an error.
Without Slots | With Slots |
---|---|
Instances can have arbitrary attributes added. | Strictly enforces a predefined set of attributes. |
Risk of accidental attribute name collisions. | Helps prevent data integrity issues. |
Limited control over the attribute set. | Provides a clear contract for class attributes. |
However, it’s important to note that using slots also has some limitations, such as the inability to add new attributes dynamically. Therefore, slots should be used judiciously based on your specific requirements.
Implementing Slots in Your Python Code
Slots are a valuable feature in Python that allow you to optimize memory usage and attribute access speed in your classes. To implement slots, you define a special attribute called __slots__ within your class. This attribute is a tuple listing the names of the attributes that instances of the class are allowed to have. Let’s explore how to use slots and the advantages they offer in detail.
class MyClass: __slots__ = (‘attr1’, ‘attr2’, ‘attr3’) |
In this example, instances of the MyClass can only have attr1, attr2, and attr3 as attributes.
Advantages of Using Slots
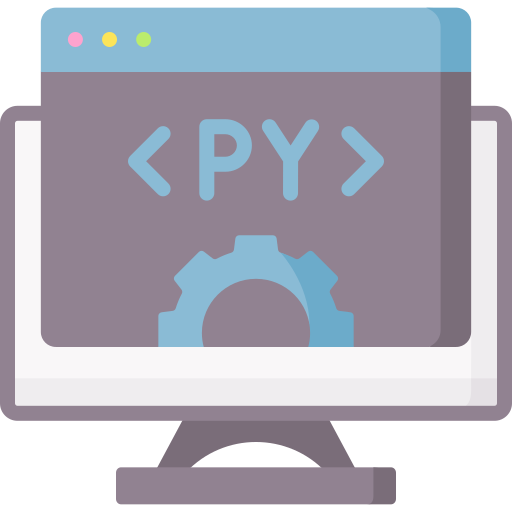
In Python, the use of slots is a technique that offers several advantages, including memory efficiency, faster attribute access, and the prevention of unwanted attributes. Let’s delve into each of these advantages, providing detailed explanations and examples where necessary.
Memory Efficiency
Memory efficiency is a crucial concern when developing programs, especially when dealing with a large number of objects. Slots can significantly reduce the memory footprint of your program by eliminating the need for a dynamic dictionary to store object attributes.
When you create a class without slots, each instance of that class has a built-in dictionary to store its attributes. This dictionary adds overhead, consuming memory and increasing the overall size of the object. However, when you use slots, this dictionary is no longer present, resulting in memory savings. Let’s illustrate this with a simple example:
class WithoutSlots: def __init__(self, name, age): self.name = name self.age = age class WithSlots: __slots__ = (‘name’, ‘age’) def __init__(self, name, age): self.name = name self.age = age |
In the above code, the WithoutSlots class uses a dictionary to store attributes, whereas the WithSlots class specifies its attributes using the __slots__ attribute. As a result, WithSlots instances use less memory because they don’t have the dictionary overhead.
Faster Attribute Access
Slots not only save memory but also improve the speed of attribute access. When you access an attribute in a class with slots, Python can directly index into a fixed, known memory location to retrieve the attribute’s value. This is in contrast to classes without slots, where a dictionary lookup is required to find the attribute. Here’s an example to demonstrate the performance difference:
import timeit class WithoutSlots: def __init__(self, name, age): self.name = name self.age = age class WithSlots: __slots__ = (‘name’, ‘age’) def __init__(self, name, age): self.name = name self.age = age obj1 = WithoutSlots(“Alice”, 30) obj2 = WithSlots(“Bob”, 25) # Measure attribute access time access_time1 = timeit.timeit(lambda: obj1.name, number=1000000) access_time2 = timeit.timeit(lambda: obj2.name, number=1000000) print(“Attribute access time without slots:”, access_time1) print(“Attribute access time with slots:”, access_time2) |
In this example, we compare the time it takes to access the name attribute in instances of both WithoutSlots and WithSlots classes. You’ll find that accessing attributes in the class with slots is faster due to the absence of dictionary lookup.
Preventing Unwanted Attributes
Using slots also helps in maintaining a clean and consistent object model by preventing the accidental addition of new attributes. When you define a class with slots, it restricts the attributes that can be assigned to an instance to only those listed in the __slots__ tuple. This restriction acts as a safeguard, reducing the chances of introducing unintended attributes. Consider the following example:
class WithSlots: __slots__ = (‘name’, ‘age’) def __init__(self, name, age): self.name = name self.age = age # Attempting to add a new attribute to an instance with slots will raise an AttributeError obj = WithSlots(“Charlie”, 35) obj.city = “New York” # This line will result in an error |
In this example, trying to add the city attribute to an instance of the WithSlots class will raise an AttributeError. This behavior ensures that the object’s structure remains consistent and that only the intended attributes are used.
Considerations and Limitations
Slots in Python offer numerous advantages, as discussed earlier, such as improved memory efficiency, faster attribute access, and the prevention of unwanted attributes. However, it’s essential to understand the considerations and limitations associated with using slots to make informed decisions when incorporating them into your code.
Inheritance Complexity
One significant consideration when using slots is dealing with inheritance. When a class defines slots, it restricts the attributes that can be assigned to instances of that class. However, when you create a subclass, Python requires you to redefine the __slots__ attribute explicitly for that subclass. This can introduce complexity when dealing with class hierarchies. Consider the following example:
class Parent: __slots__ = (‘name’, ‘age’) class Child(Parent): pass # This will raise an error because Child does not define its own __slots__ obj = Child() obj.city = “New York” |
In this example, trying to assign the city attribute to an instance of the Child class results in an AttributeError. To resolve this, you would need to define __slots__ explicitly for the Child class, potentially leading to more maintenance overhead in larger class hierarchies.
Reduced Flexibility
One of the trade-offs of using slots is reduced flexibility in terms of attribute assignment. When you define slots for a class, you explicitly list the attributes allowed. While this restriction can prevent unintended attribute creation, it can also limit the dynamic nature of Python objects.
Consider a scenario where you want to add new attributes to objects dynamically based on user input or changing requirements. With slots, this becomes challenging, as you must modify the __slots__ attribute each time you want to introduce a new attribute. This lack of flexibility may not be suitable for all use cases.
Not Always the Best Solution
While slots offer significant benefits, they may not always be the best solution for every Python application. Their advantages become most apparent in resource-intensive or performance-critical situations, where memory optimization and attribute access speed are crucial.
For small-scale applications or scripts where these concerns are less critical, the benefits of using slots may not be very noticeable. In such cases, the added complexity of managing slots and the reduced flexibility in attribute assignment might not justify their use.
It’s important to carefully assess the specific requirements and performance considerations of your project before deciding whether to employ slots.
Practical Examples
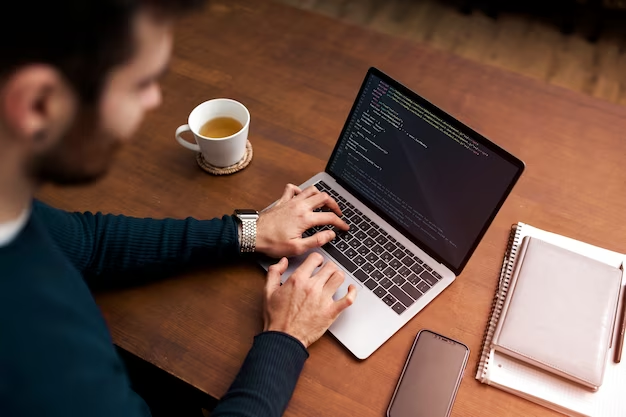
Slots in Python provide a mechanism to limit dynamic attribute creation in classes, leading to improved memory efficiency and potentially faster execution. Let’s explore practical examples to illustrate the benefits of using slots in Python.
Example 1: A Simple Class with Slots
Consider a simple class Point that represents a point in a 2D space. We’ll implement this class using slots to restrict attribute creation to only x and y. Here’s the code:
class Point: __slots__ = (‘x’, ‘y’) def __init__(self, x, y): self.x = x self.y = y |
In this example, the __slots__ attribute is defined as a tuple containing the allowed attribute names: ‘x’ and ‘y’. By doing this, we limit the object’s attributes to just these two, preventing the creation of any additional attributes. This results in a more memory-efficient class definition.
Example 2: Measuring Performance Improvements
To truly understand the advantages of using slots, we’ll compare the memory usage and speed of classes with and without slots. We’ll create two classes, WithSlots and WithoutSlots, and evaluate their memory consumption and instantiation speed.
import timeit import sys class WithSlots: __slots__ = (‘a’, ‘b’, ‘c’) class WithoutSlots: pass # Measuring memory usage print(“WithSlots memory:”, sys.getsizeof(WithSlots())) print(“WithoutSlots memory:”, sys.getsizeof(WithoutSlots())) # Measuring speed print(“WithSlots speed:”, timeit.timeit(lambda: WithSlots(), number=1000000)) print(“WithoutSlots speed:”, timeit.timeit(lambda: WithoutSlots(), number=1000000)) |
Memory Usage Comparison
In the memory usage comparison, we use the sys.getsizeof() function to determine the memory footprint of instances of the WithSlots and WithoutSlots classes. The result will show that the class with slots (WithSlots) consumes less memory compared to the class without slots (WithoutSlots). This memory optimization becomes more significant as the complexity of the class and the number of instances increase.
Speed Comparison
The speed comparison involves measuring the time taken to create 1,000,000 instances of each class using the timeit module. The result will indicate that class instantiation with slots (WithSlots) is generally faster than the class without slots (WithoutSlots). This performance improvement can be especially valuable in scenarios where objects need to be created rapidly or in large quantities.
Conclusion
Using slots in Python to limit dynamic attribute creation and improve speed is a powerful technique, especially in scenarios where resource optimization is crucial. By understanding and implementing slots effectively, you can harness this feature to make your Python programs more memory-efficient and faster.
Remember, while slots offer significant benefits in certain situations, they’re not a one-size-fits-all solution. It’s important to evaluate the specific needs and constraints of your application before deciding to use slots. With this knowledge, you’re now equipped to make an informed decision about using slots in your next Python project.
FAQs
Consider using slots when you have a large number of instances of a class and memory efficiency is critical. It’s also useful when you want to prevent dynamic creation of new attributes.
Yes, but each subclass needs to define its own __slots__. The slots of parent classes are not inherited.
The main downside is the loss of flexibility in dynamically adding new attributes to instances of the class.
The improvement can be significant in terms of memory usage, especially with a large number of objects. The speed improvement is noticeable but less dramatic.
No, attempting to add attributes not listed in __slots__ will result in an AttributeError.