In the realm of Python programming, developers frequently grapple with the challenge of managing dynamic attribute creation within classes, a task that can be both perplexing and crucial for efficient code execution. This article delves deeply into this topic, focusing particularly on the strategic use of slots as a means to restrict attribute generation during runtime. Slots in Python serve as a definitive framework for attribute management, offering a more structured and memory-efficient approach. They essentially replace the typical dynamic nature of Python objects with a static structure, limiting the creation of attributes to those explicitly declared. This mechanism is especially advantageous in scenarios where the attributes of a class are well-defined and unlikely to change, allowing for a more controlled and optimized environment.
Furthermore, the use of slots contributes to a more predictable and error-resistant coding experience. By explicitly defining the attributes that an object can possess, slots reduce the likelihood of unintended attribute creation or modification, a common source of bugs in dynamic systems. This makes the codebase more robust and easier to maintain, as the structure and behavior of objects are clearly defined and enforced by the Python interpreter.
Overall, the introduction of slots in Python programming represents a significant paradigm shift from the traditional, flexible nature of object attribute management. It offers a valuable tool for developers seeking to optimize memory usage and improve the performance of their applications, all while maintaining a high level of code integrity and reliability. As such, understanding and effectively utilizing slots can be a pivotal skill in the toolkit of any Python programmer, particularly those working on large-scale or performance-critical applications.
The Concept of Dynamic Attribute Creation
Python objects are capable of storing an indefinite number of attributes. Intriguingly, these attributes can be dynamically generated at runtime, not just at the class definition stage. Consider the class `Person`, defined as follows:
```python
class Person:
def __init__(self, name):
self.name = name
```
Using this class, one can create an instance and assign values to its attributes, as shown:
```python
me = Person('Aquiles')
print(me.name) # Outputs 'Aquiles'
```
Here, a `Person` object named `me` is created with the attribute `name` set to ‘Aquiles’. Python allows for the modification of existing attributes and the addition of new ones:
```python
me.name = 'John'
me.last_name = 'Doe'
print(me.name) # Outputs 'John'
print(me.last_name) # Outputs 'Doe'
```
This flexibility, however, is a double-edged sword. It can lead to unnoticed errors in complex objects, as demonstrated in the example of a hypothetical `Camera` class:
```python
camera = Camera()
camera.exposure_time = '5ms' # Intended attribute
camera.exp_time = '10ms' # Mistaken attribute
```
An Introduction to Slots in Python
Slots in Python present a pragmatic solution to the often uncontrolled nature of attribute creation in classes. By defining a clear boundary, slots enable developers to specify precisely which attributes an object can possess. This feature is particularly beneficial in maintaining the integrity and predictability of the object model within an application.
Consider the following example:
```python
class Person:
__slots__ = 'name'
def __init__(self, name):
self.name = name
```
In this class definition, the `__slots__` mechanism explicitly declares that objects of the `Person` class should only have a `name` attribute. The simplicity of this syntax belies its power. When an attempt is made to introduce an attribute not included in `__slots__`, Python raises an `AttributeError`:
```python
me = Person('Aquiles')
me.last_name = 'Doe' # Triggers AttributeError
```
This restriction ensures that objects of the `Person` class adhere strictly to the defined structure, preventing accidental attribute additions that could lead to bugs or memory inefficiencies. The flexibility of slots is evident when requirements change. For instance, if it becomes necessary to include a `last_name` attribute, `__slots__` can be easily modified:
```python
class Person:
__slots__ = 'name', 'last_name'
```
Now, objects of the `Person` class can legally have both `name` and `last_name` attributes:
```python
me = Person('Aquiles')
me.last_name = 'Doe' # Now allowed
```
This expanded `__slots__` definition allows for the addition of the `last_name` attribute while still maintaining the memory benefits and attribute control provided by slots.
However, the use of slots is not without its complexities. Inheritance, for instance, introduces additional considerations. A subclass that does not define `__slots__` will inherit the slots from its superclass, but it will also have a `__dict__` attribute, allowing for the dynamic addition of new attributes. Conversely, if the subclass defines its own `__slots__`, it will only have those slots, unless the `__dict__` is explicitly included in the slots definition.
Moreover, the use of slots has implications on memory usage and performance. Since slots eliminate the need for each object to have a `__dict__`, they can significantly reduce the memory footprint of an application, particularly one that instantiates many objects. This can lead to more efficient memory usage and potentially faster attribute access times. Slots offer a robust and flexible mechanism for managing attributes in Python classes. By providing clear constraints on attribute creation, slots enhance code reliability, maintainability, and performance. However, their implementation requires careful consideration, particularly in the context of inheritance and application architecture, to fully harness their benefits.
The Impact of Slots on Class Complexity
While slots control attribute creation, managing them in complex classes can be challenging. Notably, class-level attributes become read-only:
```python
class Person:
__slots__ = 'name', 'last_name'
age = 35
```
Attempting to modify such attributes results in an error:
```python
me = Person('Aquiles')
me.age = 50 # Triggers AttributeError
```
Attribute Storage: The Role of `__dict__`
Python objects store attributes in a dictionary, `__dict__`. Examining the `Person` class and its instance reveals this:
```python
print(Person.__dict__) # Shows class attributes and methods
print(me.__dict__) # Shows instance attributes
```
However, with slots, `__dict__` is absent, and attributes are directly stored in `__slots__`:
```python
class Person:
__slots__ = 'age', 'name'
print(me.__slots__) # Shows ('age', 'name')
```
Exploring Slots and Descriptors
When slots are implemented in Python classes, they are internally treated as descriptors, a concept pivotal to understanding the behavior of attributes defined within slots. Descriptors are special objects that customize attribute access, and in the case of slots, they play a crucial role in managing how attributes are accessed and modified.
Descriptors provide a protocol for attribute access, offering methods to get, set, and delete an attribute. In the context of slots, each attribute named in the `__slots__` declaration becomes a descriptor. This is why attempting to access or modify an attribute not defined in `__slots__` triggers an `AttributeError`. The descriptor protocol ensures that only the attributes explicitly declared in `__slots__` are accessible, thereby enforcing the constraints imposed by slots.
This treatment of slot attributes as descriptors explains why certain attributes become read-only when slots are used. For instance, if an attribute is defined only in the `__slots__` of a parent class and not in a subclass, the descriptor for that attribute does not include a setter method in the subclass. Consequently, the attribute becomes read-only in instances of the subclass. This behavior underlines the importance of carefully designing class hierarchies and slot declarations to avoid unintended read-only attributes. Understanding that slots employ descriptors for attribute management is essential for developers working with Python. This knowledge provides insight into the internal workings of slots and their impact on attribute behavior. It equips developers with the ability to predict and control how attributes behave in their classes, leading to more robust and predictable code. Moreover, this understanding is critical when designing classes for inheritance, ensuring that attributes behave as expected in both parent and child classes.
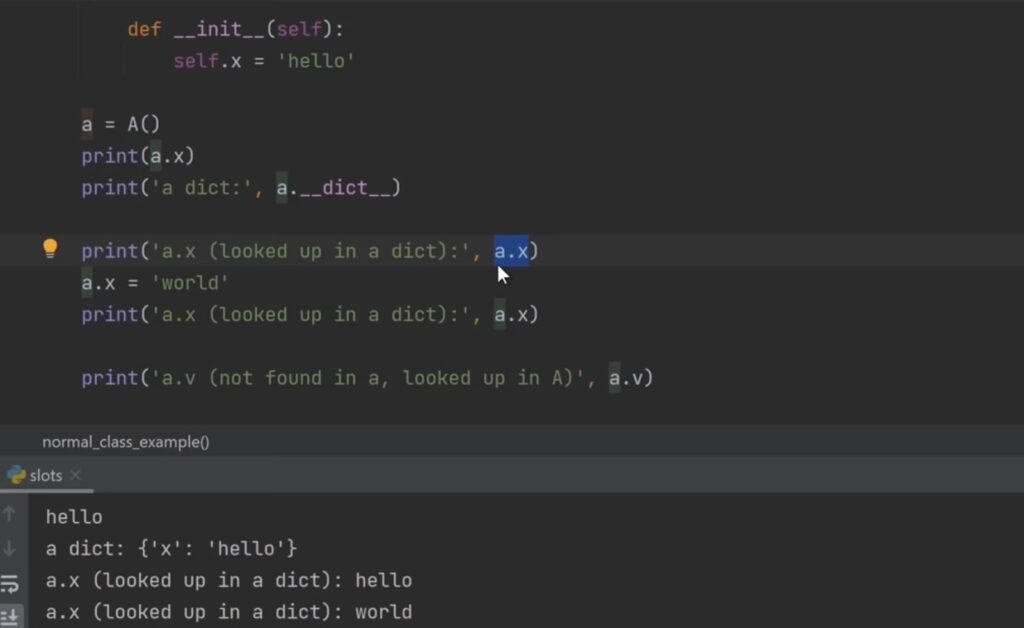
Hybrid Class: Combining Slots and `__dict__`
By including `__dict__` in `__slots__`, a hybrid class is created, allowing both slotted and dynamic attributes:
```python
class Person:
__slots__ = 'age', 'name', '__dict__'
me = Person(35, 'Aquiles')
me.new_var = 10
print(me.__dict__) # Outputs {'birth_year': 1986, 'new_var': 10}
```
This article has explored the intricacies of dynamic attribute management in Python, highlighting the use of slots and their implications on class design and behavior.
Exploring Slots in Inheritance
This section delves into the nuanced interaction of slots with inheritance in Python, shedding light on their behavior and the consequential implications for object-oriented programming. Inheritance, a cornerstone of Python’s class mechanism, allows for the creation of subclasses that inherit properties and behaviors from parent classes. However, when slots are introduced into this mix, their behavior and impact need careful consideration.
When a class defined with slots is inherited, the subclass inherits these slots, but the way it handles additional attributes can vary based on its own definition. If the subclass does not define its own `__slots__`, it inherits the slots from the parent but also retains the flexibility of the standard object model, including a `__dict__` for dynamic attribute assignment. This dual behavior enables subclasses to benefit from the memory efficiency of slots while still allowing for dynamic attribute creation.
On the other hand, if a subclass defines its own `__slots__`, it will only possess the slots explicitly declared in its scope, along with those inherited from the parent class. This can lead to a more restricted attribute set, emphasizing the need for careful planning when designing class hierarchies involving slots. The subclass’s slots do not automatically include a `__dict__`, implying that attributes not defined in the slots cannot be dynamically added.
Furthermore, the combination of slots and inheritance can lead to complex scenarios, such as read-only attributes or conflicts between the parent’s and child’s slots. These complexities require developers to have a deep understanding of both inheritance and slots to effectively utilize them in class design. Understanding the interaction between slots and inheritance is vital for crafting efficient, robust, and maintainable Python code, especially in applications where memory management and attribute control are paramount.
Inheriting from a Class with Slots
Consider a class `Person` with defined slots and a subclass `Student` inheriting from it:
```python
class Person:
__slots__ = 'age', 'name'
def __init__(self, age, name):
self.age = age
self.name = name
class Student(Person):
def __init__(self, age, name, course):
super(Student, self).__init__(age, name)
self.course = course
```
In the above scenario, `Student` inherits `Person` but does not declare its own slots. It behaves like a typical class, supporting dynamic attribute creation and retaining access to its parent’s slots:
```python
me = Student(35, 'Aquiles', 'Physics')
print(me.__dict__) # Displays {'course': 'Physics'}
print(me.__slots__) # Shows ('age', 'name')
```
The `Student` class, therefore, inherits the slots from `Person` but can also define additional attributes dynamically.
Defining Slots in the Child Class Only
A contrasting scenario arises when the parent class does not define slots, but the child class does:
```python
class Person:
def __init__(self, age, name):
self.age = age
self.name = name
class Student(Person):
__slots__ = 'course'
```
This structure allows for dynamic attributes in both the parent and child classes:
```python
me = Student(35, 'Aquiles', 'Physics')
print(me.__dict__) # Shows {'age': 35, 'name': 'Aquiles'}
```
In this case, the presence of `__dict__` in either the parent or child class enables dynamic attribute creation.
Slots in Both Parent and Child Classes
When both the parent and the child classes define slots, the resulting behavior aligns with the expected characteristics of slots:
```python
class Student(Person):
__slots__ = 'course'
# ...
me = Student(35, 'Aquiles', 'Physics')
me.new_var = 10 # Triggers AttributeError
```
Here, the `Student` class restricts attribute creation strictly to those defined in its slots.
Impact of Slots on Performance and Memory Usage
Slots were introduced in Python primarily to enhance attribute access speed and reduce memory usage. They can significantly improve performance, especially when dealing with a large number of objects, as demonstrated by frameworks like SQLAlchemy.
Concluding Thoughts on Using Slots
In the intricate landscape of Python programming, slots emerge as a compelling feature, particularly for developers who seek to optimize both memory usage and attribute access speed. This article has illuminated the multifaceted nature of slots, especially in the context of inheritance, providing insights into how they interact with Python’s class hierarchy. One of the pivotal advantages of slots is their ability to significantly reduce memory footprint. In scenarios where a program generates a multitude of objects, the memory savings from slots can be substantial. This aspect is particularly beneficial in large-scale applications, like data processing frameworks or complex web applications, where efficient memory management is crucial. For instance, the use of slots in the SQLAlchemy framework underlines their effectiveness in real-world applications, showcasing measurable improvements in memory efficiency.
Additionally, slots can enhance attribute access speed. While the performance gain might not be noticeable in smaller scripts or applications, it becomes more evident in systems where attribute access is a frequent operation. Therefore, when designing high-performance applications, especially those requiring rapid access to object attributes, considering slots becomes a prudent choice.
However, it’s important to approach the use of slots with a nuanced understanding. Improper implementation, especially in the context of inheritance, can negate the benefits. Careless inheritance can inadvertently introduce inefficiencies, particularly when subclasses ignore or improperly use the slots mechanism. Developers must, therefore, be judicious in their application of slots, ensuring that their use aligns with the overall architectural and performance goals of the application. Moreover, while slots offer a straightforward solution to restrict dynamic attribute creation, they are not a panacea for all design challenges in Python. In cases where flexibility in attribute management is desired, or where the memory and performance benefits are marginal, the traditional use of `__dict__` may still be preferred. The decision to use slots should be guided by the specific requirements of the project, balancing the need for efficiency with the flexibility and maintainability of the code.
In conclusion, slots in Python serve as a powerful tool in a developer’s arsenal, offering significant benefits in memory optimization and attribute access speed. However, their effectiveness is contingent upon thoughtful implementation and a clear understanding of their interaction with Python’s class structure and inheritance model. As with any tool, the key lies in using slots judiciously, tailoring their application to the specific needs and constraints of each individual project.