In the journey of learning programming, one often stumbles upon advice highlighting the crucial role of adding comments to code. Comments serve as a window into the developer’s thought process at the time of coding and may offer hints on potential improvements. This practice becomes even more vital in environments where personnel changes are frequent, as comments and documentation greatly facilitate the onboarding process for new developers.
Python, known for its robustness, extends this utility to its system for documenting functions, classes, and variables. The language’s documentation capabilities are so comprehensive that they allow for interactive help in the console, enable testing, and can even be transformed into aesthetically pleasing HTML or PDF formats. This tutorial aims to guide readers through the process of writing effective documentation for Python projects and hosting it on Read The Docs, a free platform.
Constructing a Basic Python Module
The tutorial begins by guiding readers through the creation of a basic Python module, starting with the establishment of a Virtual Environment. The initial step involves creating a project folder and within it, a subfolder named `my_module`, containing an empty `__init__.py` file. The next phase involves crafting a `factorial.py` file with the following code:
```python
import math
def factorial(n):
# Implementation of factorial function
```
This example, available under tag 0.1 on GitHub, demonstrates a practical use-case:
```python
>>> from my_module.factorial import factorial
>>> factorial(5)
120
```
The interactive help feature can also be utilized:
```python
>>> help(factorial)
```
While the initial help output for `factorial` may be sparse, the tutorial promises to delve deeper into enhancing its descriptiveness. Through this step-by-step approach, the tutorial aims to equip Python programmers with the tools and knowledge necessary to create well-documented and maintainable code, an essential skill in the ever-evolving landscape of software development.
Enhancing Code Understanding with Docstrings in Python
When delving into the world of programming, particularly for beginners, understanding the importance of comments in code is fundamental. Comments are more than just annotations; they are a window into the developer’s thought process, offering explanations and insights that the code alone cannot convey. They provide context, explain the purpose of certain blocks of code, and can even suggest areas for potential improvement. This aspect of code commenting becomes particularly critical in environments where development teams are fluid, and personnel changes are frequent.
In such dynamic environments, detailed comments and comprehensive documentation become indispensable tools for onboarding new developers. They enable newcomers to quickly adapt to the existing codebase, understand the rationale behind specific implementations, and maintain the continuity of the project. Good documentation acts as a guide, reducing the learning curve and ensuring that even complex systems can be understood and managed by those who weren’t involved in their initial development. Python, in particular, stands out for its robust system of documenting functions, classes, and variables. One of Python’s strengths is its readability and the emphasis it places on clear, concise code. This philosophy extends to its documentation practices as well. Python supports writing docstrings — detailed explanations written directly in the source code that describe what a function or class does, its parameters, return values, and other important aspects.
These docstrings are versatile; they not only serve as in-code documentation but can also be accessed interactively through the console. Python’s rich ecosystem includes tools like Sphinx, which can transform these docstrings into well-structured, visually appealing documentation in formats like HTML or PDF. This feature is particularly useful for creating online documentation that can be easily shared and accessed.
This tutorial aims to guide developers through the process of creating comprehensive documentation for their Python projects. It covers the best practices for writing effective docstrings and comments, using Sphinx to generate documentation, and demonstrates how to host this documentation for free on Read The Docs. By following these guidelines, developers can ensure their projects are well-documented, thereby enhancing collaboration, maintainability, and overall project success.
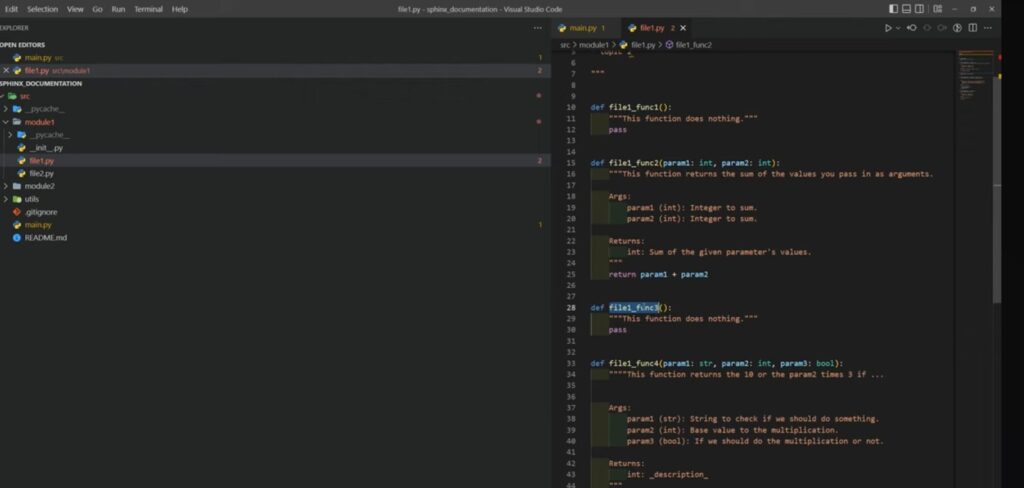
Crafting a Basic Python Module for Tutorial Purposes
The tutorial begins with the construction of a simple Python module, an excellent starting point for understanding both Python programming and documentation practices. It’s highly recommended for developers to work within a Virtual Environment. This isolated environment allows for better management of dependencies and packages, ensuring that the project does not interfere with the system-wide Python installation or other Python projects.
To set the foundation for this tutorial, developers are advised to create a project folder. This folder will serve as the workspace containing all the necessary files for the module. Within this project folder, a subfolder named `my_module` should be created. The `my_module` folder will represent the Python module. Inside this subfolder, an `__init__.py` file is needed. This file is crucial as it signifies to Python that this directory should be treated as a Python module. The presence of an `__init__.py` file, even if it’s empty, allows for the contents of the folder to be imported as a module.
Next, the creation of a `factorial.py` file within the `my_module` folder is required. This file will contain specific code to calculate the factorial of a number, a common example used in programming tutorials. The factorial function is not only straightforward but also provides a good opportunity to demonstrate how functions work and how they can be documented effectively. An important note for developers: when making changes to a Python module, simply reimporting the module in the Python interpreter or script won’t reflect the latest changes. This is because Python caches imported modules and does not automatically reload them on reimport. To see the updated functionalities, one must quit the interpreter or script and reimport the module after restarting it. This step ensures that the latest version of the module, with all recent changes, is being used. This aspect is crucial during development and testing, as it ensures that the code being tested is the most current version.
Implementing Docstrings for Improved Clarity
The use of Python’s `help` command searches for a string immediately following a function’s definition. For instance, initially, the `factorial` function lacked a descriptive string. By adding a docstring, the function becomes more informative:
```python
def factorial(n):
'Function to calculate the factorial'
# ...
```
Python traditionally uses triple quotes for docstrings, allowing for multiline descriptions and examples. The inclusion of examples in the docstring enhances the help command’s output, providing a clearer understanding of the function’s purpose and usage.
Extending Documentation Beyond Functions
Python’s capability to document entire modules is a feature that significantly enhances the readability and maintainability of code, especially in larger projects with multiple modules. By placing a descriptive docstring at the beginning of a module file, developers can provide a concise yet comprehensive overview of the module’s purpose, functionalities, and any other relevant information. This practice is not just a recommendation but a standard in Python’s community for writing clean and understandable code.
Consider the following example where a descriptive string is placed at the top of the `factorial.py` file:
```python
"""
Module factorial
================
This module supplies one function, factorial() to calculate the factorial of an integer.
"""
import math
# ...
```
In this example, the docstring succinctly describes the primary functionality of the module, i.e., to provide a function for calculating the factorial of an integer. This kind of documentation at the module level is highly beneficial for several reasons:
- Clarity and Overview: It gives anyone reading the code a quick overview of what the module does without delving into the specifics of the code. This is particularly useful in larger projects where understanding the purpose of each module at a glance can save significant time;
- Navigation Aid: For developers navigating through a package full of various modules, these docstrings serve as signposts, guiding them to the correct module for their needs. This is especially helpful when working in collaborative environments or in open-source projects where the codebase may be accessed by many different contributors;
- Command Line and Interactive Help: When using Python’s interactive console or command line, these module-level docstrings can be accessed via help commands. For instance, typing `help(‘my_module.factorial’)` would display the docstring, providing immediate, in-context documentation for users and developers;
- Generating Documentation: Tools like Sphinx can use these docstrings to automatically generate well-structured and navigable HTML (or other formats) documentation. This automated process streamlines the creation of user-friendly documentation, making the module more accessible to a broader audience.
Docstrings at the module level, therefore, are a valuable tool for both the code author and other developers. They contribute significantly to the understanding of the module’s intent and capabilities, whether viewed directly in the code, through an IDE, or via command line tools. This practice is a hallmark of well-documented, professional Python code and is highly encouraged for developers aiming to write clear, maintainable, and accessible code.
Transitioning from Docstrings to Doctest
Testing is a fundamental aspect of collaborative coding. Doctest in Python leverages examples provided in docstrings to test code functionality. By running the doctest command, Python executes the examples and verifies if the output matches the expected results. This feature is particularly useful for validating basic functionalities of packages.
Documenting Classes and Methods
Docstrings in Python are not limited to just modules; they are equally applicable and valuable when used in classes and methods. This practice plays a vital role in maintaining clarity and promoting a deeper understanding of the code, especially in more complex projects with numerous classes and methods. The application of docstrings to classes and methods aids significantly in self-documenting the code, making it more readable and maintainable.
Consider a Python file, `people.py`, containing classes like `Person` and `Teacher`. Each of these classes, along with their methods, can have its own docstring that details its purpose, usage, and any other relevant information. For instance:
```python
class Person:
"""
Class Person
------------
Represents a generic person.
Attributes:
name (str): The name of the person.
age (int): The age of the person.
"""
def __init__(self, name, age):
"""
The constructor for Person class.
Parameters:
name (str): The name of the person.
age (int): The age of the person.
"""
self.name = name
self.age = age
class Teacher(Person):
"""
Class Teacher
-------------
Represents a teacher, which is a type of Person.
Attributes inherited from Person:
name (str): The name of the teacher.
age (int): The age of the teacher.
"""
# Methods with their own docstrings...
```
In this example, each class and its constructor (`__init__` method) have docstrings that explain what the class is for, its attributes, and how to use it. This level of documentation is crucial, especially in larger projects with many classes and methods. It ensures that any developer, whether new to the project or returning after some time, can quickly understand the purpose and functionality of each class and method without needing to decipher the code’s logic.
Effective use of docstrings in classes and methods not only aids in understanding and maintaining the code but also facilitates the generation of comprehensive external documentation, making the project more accessible and user-friendly. This documentation practice is a hallmark of professional Python programming and greatly contributes to the overall quality and sustainability of software projects.
Building Comprehensive Documentation with Sphinx
Once a package is developed with each module and function documented, Sphinx can compile all docstrings into a cohesive set of documentation, such as a website or a PDF. Sphinx transforms files written in RestructuredText into various formats, allowing for the creation of a centralized, easily navigable documentation source.
Integrating Module Documentation with Sphinx
Sphinx can automatically generate documentation for modules. The process involves updating the Sphinx configuration file and creating specific `.rst` files for each module. By linking these files in the main documentation index, a comprehensive and accessible set of documentation is produced, showcasing the depth and functionality of the project.
The tutorial encapsulates the journey from writing basic docstrings to utilizing Sphinx for creating elaborate documentation. It underscores the importance of well-documented code in enhancing understandability and facilitating collaboration, especially in dynamic development environments.
Documentation Pages for Enhanced Clarity
In their pursuit of effective documentation, developers have the flexibility to go beyond the automatic `automodule` command and `:members:` option in Sphinx. They can craft documentation for specific elements, enriching it with more detail than what’s provided in the docstrings. Let’s explore how to refine the `factorial.rst` file by adding comprehensive content.
Expanding the factorial.rst File with Detailed Information
The `factorial.rst` file is updated to include a more descriptive section about calculating factorials, incorporating examples and explanations that extend beyond the code’s basic functionality:
```rst
How to calculate the factorial
==============================
Description and example of factorial calculation.
.. automodule:: my_module.factorial
```
When the documentation is rebuilt, these changes are reflected in the output, adding depth and structure to the content. The tutorial doesn’t aim to cover all aspects of Restructured Text but highlights the importance of formatting with titles and subtitles using ‘=’, ‘-‘ and so on.
Incorporating Code Examples in Documentation
When including code examples in documentation, developers have options like directly writing the example or using the `code-block` directive for better formatting and clarity:
```rst
Example of factorial function usage:
.. code-block:: pycon
>>> factorial(5)
120
```
This approach allows for a more detailed and instructional style of documentation, transforming it into a comprehensive resource that extends beyond mere code explanation.
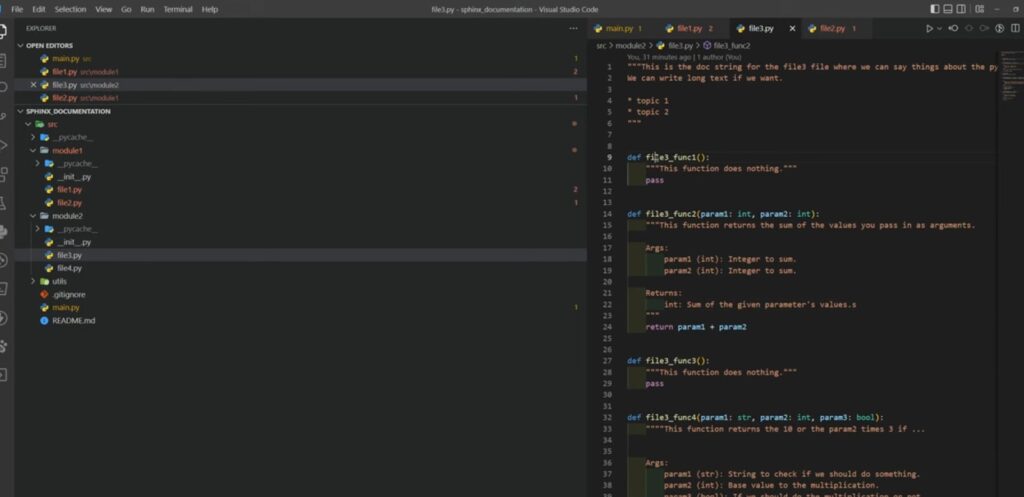
Styling Docstrings for Detailed Explanation
In enhancing docstrings, developers can include detailed descriptions of parameters and return types. This specificity aids in understanding the function’s input and output, thereby reducing potential errors. Various styles can be adopted for this, like reST, Epydoc, Google, or Numpy style. The choice depends on the developer’s preference, as they all ultimately serve the same purpose of providing clear and detailed documentation.
For instance, the `factorial` function in `factorial.py` is updated with detailed parameter and return type information:
```python
def factorial(n):
"""
Detailed documentation of the factorial function, including parameter types and return type.
"""
```
Rebuilding the documentation incorporates these details into the `factorial.html` page, enhancing the information’s comprehensiveness and accessibility.
Linking Between Modules in Documentation
Cross-referencing within documentation is crucial, especially when classes or functions are interdependent. For example, in the `people.py` file, linking the `Teacher` class to the `Person` class it subclasses makes the documentation more interconnected and user-friendly:
```python
class Teacher(Person):
"""Class documentation, linking to the :class:`Person`."""
```
This link is reflected in the built documentation, providing a seamless navigation experience across different modules or classes.
Running Tests with Sphinx for Reliable Documentation
Integrating doctests into Sphinx allows for simultaneous documentation building and code testing. This process ensures that the documentation is up-to-date and accurate with the code’s current state. To enable doctests in Sphinx, the `doctest` extension is added to the `conf.py` file, and Sphinx is triggered with the doctest build option.
In some cases, additional setup like explicit imports may be required for doctests to function correctly. Incorporating these imports into the docstrings ensures that the examples are self-contained and testable:
```python
def factorial(n):
"""
Example with explicit import for doctest.
"""
```
Running doctests as part of the documentation process enhances the quality of both the code and its accompanying documentation, providing a more robust and reliable resource for developers.
Throughout this tutorial, from customizing documentation pages to integrating tests, the aim is to illustrate the versatility and importance of thorough documentation in Python. By following these steps, developers can create detailed, accessible, and reliable documentation that enhances the overall quality and usability of their code.
Hosting and Customizing Documentation with Read The Docs
After mastering the creation of documentation locally using Sphinx, developers might consider making their documentation accessible to a broader audience. Hosting documentation online not only aids others in learning from one’s code but also enhances online visibility, a common practice among significant projects.
Hosting Documentation Online: The Practical Approach
Hosting documentation on a personal web server is certainly an option for many developers, but it comes with its own set of challenges, including additional costs and the effort required to maintain and update the pages. For individual developers or small teams, these challenges can be significant, diverting valuable time and resources away from the core development work. This is where Read The Docs (RTD) offers a valuable alternative solution.
Read The Docs is a popular platform designed specifically for hosting documentation. It stands out because it automates many of the tasks associated with documentation hosting, making the process more efficient and manageable, particularly for open-source projects. The platform is not only free to use, but it also streamlines the process of building and publishing documentation, offering several key advantages:
- Automatic Building: RTD automatically builds documentation from source files stored in online repositories like GitHub, GitLab, or Bitbucket. Whenever changes are pushed to the repository, RTD triggers a new build, ensuring that the documentation always reflects the latest version of the code;
- Version Control: RTD integrates seamlessly with version control systems, making it easy to manage documentation for different versions of a project. This is particularly useful for projects that maintain multiple active versions;
- Format Flexibility: RTD supports Sphinx, a powerful documentation generator, which allows for the creation of well-structured and visually appealing documentation in various formats like HTML, PDF, and ePub;
- Ease of Access: Once hosted on RTD, documentation becomes easily accessible to a wide audience via a dedicated URL. This accessibility is crucial for open-source projects seeking to reach a broad user base;
- Community and Support: RTD has a large community of users and contributors, providing a wealth of resources, guides, and support, which can be particularly beneficial for those new to documentation hosting.
By choosing Read The Docs for hosting, developers and teams can significantly reduce the overhead associated with maintaining a personal web server for their documentation. RTD’s automated processes and integration with version control systems ensure that the documentation is always up to date and in sync with the project’s progress, making it an ideal choice for projects of all sizes.
Setting Up on Read The Docs
To use Read the Docs (RTD), the first requirement is to have your documentation’s code stored in a repository on platforms such as GitHub, GitLab, or Bitbucket. This setup ensures that your documentation is version-controlled and easily accessible.
After setting up your repository, the next step involves creating an account on Read the Docs. This process is straightforward and similar to signing up for other online services. Once you have signed up, you’ll need to activate your account via an email confirmation link. This is a standard security measure to verify your email address and to prevent spam accounts.
Once your RTD account is active, you can connect it to your chosen code hosting service. This integration allows RTD to access your documentation repository. Connecting your service to RTD typically involves authorizing RTD in your code hosting platform. This authorization is necessary for RTD to automatically fetch updates from your repository and build your documentation. In cases where the desired service cannot be directly connected to RTD, there’s also an alternative option to import your repository manually. This process might involve providing the URL to your repository and some additional configuration settings to ensure that RTD can correctly build and display your documentation.
After successfully linking your repository with RTD, you can configure your documentation settings within RTD. This includes specifying which branch to build from, setting up different versions of the documentation if necessary, and customizing the build process according to the needs of your project. Using RTD involves a few simple steps: storing your documentation in a compatible repository, creating and activating an RTD account, connecting or importing your repository to RTD, and configuring your documentation settings. This setup leverages the strengths of version control and continuous integration to keep your documentation up-to-date and accessible to your audience.
Configuring Documentation Build on RTD
Once the project is imported into RTD, it’s crucial to configure the documentation build settings. This includes ensuring that the package is installed within a Virtual Environment. Sphinx, the tool used for building documentation, imports modules to access docstrings. If the module depends on packages not installed by default (like numpy), the import process might fail. A `requirements.txt` file helps RTD install all necessary packages in a virtual environment.
Another key setting is the location of the `conf.py` file, which typically resides in `docs/source/conf.py`. Users should also select the appropriate Python version for their program.
Managing Documentation Versions
RTD allows users to specify which versions of their documentation to build. This flexibility ensures that users don’t access documentation for code versions that are still in progress or not yet released, avoiding potential confusion due to discrepancies between code and documentation.
Automating Documentation Builds
Upon triggering a build in Read the Docs (RTD), users gain access to a comprehensive build history and detailed results. This history is crucial for tracking the evolution of your documentation over time. Each entry in the build history provides valuable insights, including the time of the build, the specific commit that triggered it, and the outcome of the build process. One of the key features of this build history is its ability to display any errors or issues that occurred during the build. This is particularly helpful for diagnosing problems in your documentation’s code or configuration. The error logs are detailed, offering specific information about what went wrong and where, which is essential for troubleshooting. Users can analyze these logs to identify syntax errors, broken links, or configuration issues that might have led to a failed build.
For successful builds, RTD provides an online version of the documentation. This is a sleek, web-based interface that presents your documentation in a user-friendly and accessible format. The online documentation is typically generated in HTML, though RTD also supports other formats like PDF and ePub. This versatility ensures that your documentation is accessible to a wide audience with different preferences and needs.
To keep the documentation automatically updated with each change in the repository, setting up a webhook is essential. A webhook is a powerful tool that notifies RTD of any updates in your repository, such as new commits or merged pull requests. Once notified, RTD automatically triggers a new build of the documentation. This ensures that the documentation remains synchronized with the latest code changes, providing users with the most current information.
The process of setting up a webhook typically involves a few steps within your code hosting platform. You need to specify the RTD server as the webhook’s recipient and configure the events that will trigger the webhook, such as pushes to the repository or pull request merges. Once configured, the webhook becomes an integral part of your continuous integration workflow, seamlessly keeping your documentation in line with your codebase. RTD’s build history and results feature, coupled with the webhook mechanism for automatic updates, create a dynamic and efficient system for managing documentation. This system ensures that your documentation is not only accurate and up-to-date but also easily accessible and user-friendly, enhancing the overall experience for both the documenters and the end-users.
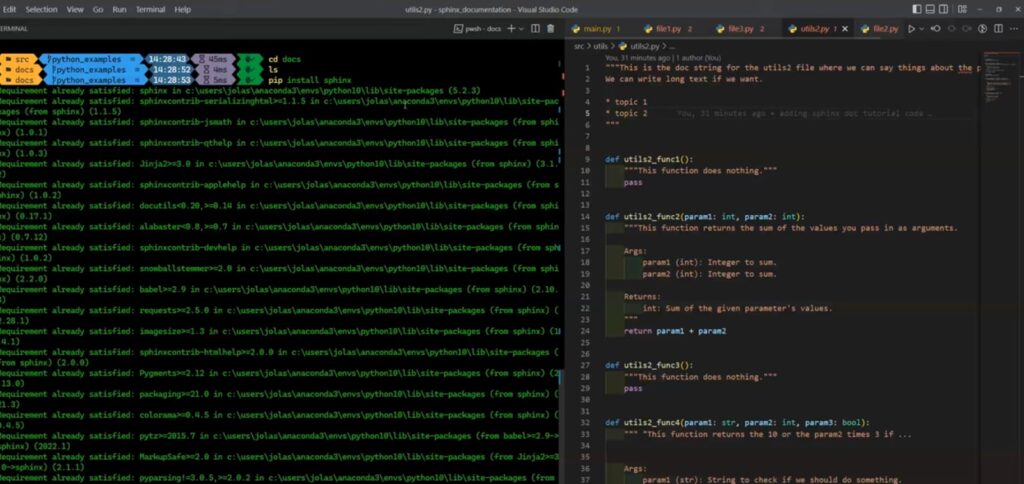
Enhancing Documentation Impact
Having documentation available as a website significantly broadens its accessibility and influence. This approach is particularly advantageous as it leverages the vast reach of the internet, making the information readily available to a global audience. With the documentation hosted online, users can access it from anywhere, at any time, without the need for downloading or specific software, except for a web browser. This ease of access is a key factor in increasing the documentation’s reach and impact.
One of the major benefits of web-based documentation is its visibility to search engines. Search engine indexing plays a crucial role in improving the discoverability of the content. When your documentation is indexed by search engines like Google, Bing, or DuckDuckGo, it becomes searchable and can be easily found by users looking for information related to your project or topic. This visibility is particularly important in the current digital age where most people turn to search engines for answers. Moreover, the web format allows for the inclusion of rich media elements like examples, images, videos, and comprehensive guides.
These elements greatly enhance the user experience by providing a more interactive and engaging way of learning. For instance, complex concepts can be illustrated through diagrams or screenshots, making them easier to understand. Additionally, code examples can be included to demonstrate practical applications, which is especially beneficial for technical documentation.
This rich, multimedia approach not only attracts attention but also facilitates quick understanding, especially for new users who might be unfamiliar with the subject matter. The ability to visually represent information, coupled with well-structured, searchable content, makes it easier for users to find what they need and comprehend it quickly. This is a significant advantage in an era where user attention spans are short, and the demand for quick, easy-to-digest information is high. Furthermore, web-based documentation allows for easy updates and revisions, ensuring that the content remains current and relevant. Changes can be made swiftly, and updates are immediately available to users, unlike printed materials which require a new edition for every update. Hosting documentation as a website offers numerous advantages. It significantly increases the documentation’s reach and impact, improves discoverability through search engine indexing, and enhances the user experience by allowing for the inclusion of examples, images, and comprehensive guides. This approach is not only attractive to users but also crucial for effective knowledge dissemination in today’s fast-paced, digitally-driven world.
Concluding Thoughts on Effective Documentation
Effective documentation is indeed a cornerstone of sustainable programming, playing a crucial role in the longevity and usability of software projects. The foundation of good documentation lies in the practice of writing clear and concise comments and docstrings within the code itself. These comments and docstrings are essential for anyone who interacts with the code, be it the original developers, collaborators, or future maintainers. They provide immediate insight into the purpose and functionality of modules, functions, and classes, making the codebase more approachable and maintainable.
Integrating tools like Sphinx takes documentation to the next level. Sphinx is a powerful documentation generator that converts these comments and docstrings into well-organized and visually appealing web pages. This transformation allows for the creation of engaging and informative websites that serve as comprehensive guides for the software. Sphinx supports various markup languages and comes with a range of extensions, enabling the inclusion of technical content, such as code snippets, graphs, and tables, in an easily digestible format. Hosting the generated documentation on platforms like Read the Docs (RTD) further simplifies the process of maintaining and distributing it. RTD provides a streamlined way to keep the documentation continuously updated, synchronized with the latest code changes, and accessible to a wide audience. Its integration with version control systems ensures that the documentation evolves alongside the software, reflecting the most current information.
This comprehensive approach to documentation – starting from in-code comments and docstrings, leveraging Sphinx for web-based documentation, and utilizing RTD for hosting and maintenance – ensures that software programs are not only functional but also understandable. It aids in making the software discoverable by a wider audience, facilitating its use, collaboration, and potential contributions from others in the programming community. By prioritizing effective documentation, developers can greatly enhance the sustainability and impact of their programming projects.