Monkey patching in Python has always been a topic of both fascination and caution among developers. At its core, monkey patching is about modifying or extending code at runtime, often leading to some surprising and powerful outcomes. This article delves into the intricacies of Python monkey-patching, its potential consequences, and best practices.
What is Monkey Patching?
In Python, monkey-patching refers to the dynamic (or runtime) modification of a class or module. In simple terms, you’re patching up the existing code with your own modifications or additions. This technique can be incredibly useful, but it’s also fraught with risks if not done carefully.
How Does it Work?
Monkey patching in Python operates by either altering or introducing new methods or attributes to pre-existing classes or modules. This is accomplished by the straightforward act of assigning fresh functions to existing attributes or methods. Below is a detailed example of monkey patching in Python:
# Example of monkey patching class MyClass: def method_a(self): return “Original method_a” def new_method_a(self): return “Patched method_a” # Applying monkey patching MyClass.method_a = new_method_a |
In this illustration, we have a class named MyClass that contains a method named method_a. Subsequently, we define a new method called new_method_a. The key aspect of monkey patching is evident when we apply it – method_a of MyClass is replaced with the new implementation, new_method_a.
Now that we have a foundational understanding of monkey patching, let’s delve into its nuances and explore best practices for its application.
Key Points to Remember:
- Monkey patching is a runtime technique for altering or extending Python code;
- It involves adding, modifying, or replacing methods and attributes;
- Careful consideration is required to mitigate potential risks.
It is vital to utilize monkey patching judiciously, considering the advantages and risks associated with it. When done correctly, it can be a valuable tool in your Python development arsenal, providing flexibility and adaptability in a variety of scenarios.
Potential Consequences of Monkey Patching
Monkey patching in Python is a dynamic and controversial programming technique. It involves changing modules or classes during runtime, effectively altering the behavior of existing code without touching its original structure. This approach is often used for quick fixes, adapting libraries for specific needs, or during testing. Despite its utility in certain scenarios, monkey patching is frequently debated due to its potential to introduce instability and maintenance challenges in software projects.
Pros of Monkey Patching
Monkey patching shines in scenarios that demand high flexibility and adaptability in code behavior. It empowers developers to tweak, extend, or fix issues in third-party libraries or existing codebases without waiting for official patches or updates. This can be especially crucial in fast-paced development environments or when dealing with legacy systems where modifying the original source is not viable. In testing, monkey patching is invaluable for simulating various states or behaviors, allowing for comprehensive testing without complex setup or dependency management.
- Dynamic code modification at runtime enhances flexibility and responsiveness to changing requirements;
- Facilitates testing by allowing the simulation of different scenarios and behaviors without altering the actual codebase;
- Enables quick fixes and adaptations of third-party libraries without needing to access or modify the original source code.
Key Point | Detailed Description |
Dynamic Flexibility | Code can be adapted on-the-fly to meet new requirements or fix issues. |
Testing and Simulation | Simplifies the creation of test scenarios by altering behavior dynamically. |
Third-party Adaptation | Allows for the customization of external libraries to fit specific project needs. |
Cons of Monkey Patching
The dark side of monkey patching lies in its potential to create maintenance nightmares, unpredictable behavior, and compatibility issues. Code that relies on monkey patching can become confusing and difficult to understand, especially for new team members or when revisiting the code after some time. It also risks introducing bugs and inconsistencies, as other parts of the application might not be aware of the modified behavior. Furthermore, updates to the original codebase can lead to significant compatibility problems, rendering the monkey-patched solutions obsolete or dysfunctional.
- Increases maintenance difficulty, leading to code that is confusing and challenging to work with;
- Risks introducing bugs and inconsistencies, especially in large and complex codebases;
- Creates potential compatibility issues with future updates to the original code, leading to a higher risk of system failures.
Issue | Detailed Impact |
---|---|
Maintenance Challenges | Makes codebases more complex and difficult to maintain, increasing the risk of errors. |
Risk of Bugs | Altered behavior may conflict with other parts of the system, leading to unexpected bugs. |
Compatibility Concerns | Future updates to the patched libraries or modules can break the custom modifications, leading to system instability. |
Real-World Applications
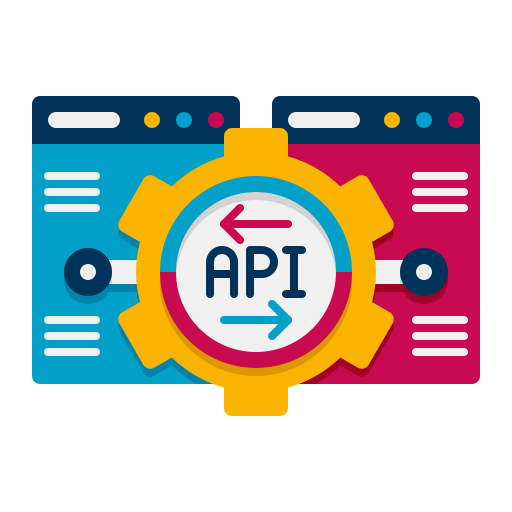
Python monkey-patching finds its utility in various scenarios:
Unit Testing
Monkey-patching is often employed in unit testing to isolate specific parts of the code for testing purposes. By temporarily replacing or modifying methods or functions, developers can control the behavior of objects or modules within the testing environment without altering the original codebase. This helps in creating reliable and isolated test cases.
Consider a scenario where you are testing a class that relies on an external service, such as a database connection. Instead of connecting to the actual database during testing, you can monkey-patch the database connection function to return mock data, ensuring that your tests are consistent and repeatable.
import database_module def mock_database_connection(): return MockDatabase() def test_my_class(): monkeypatch.setattr(database_module, ‘connect_to_database’, mock_database_connection) # Perform unit tests on your class |
Mocking External Services
Monkey-patching can also be used to mock external services, APIs, or web requests during testing. This ensures that tests can be executed without making actual network calls, improving test speed and reliability.
Let’s say your application interacts with an external REST API for data retrieval. By monkey-patching the HTTP requests library to return predefined responses, you can simulate different API responses and test your code’s behavior under various conditions.
import requests def mock_api_request(url): return MockApiResponse() def test_my_function(): monkeypatch.setattr(requests, ‘get’, mock_api_request) # Write tests for your function |
Temporary Bug Fixes
In cases where a third-party library or module contains a critical bug that affects your application, monkey-patching can provide a temporary workaround until an official patch or update is released. This allows you to mitigate the issue without waiting for the library maintainers to fix it.
Suppose you are using a third-party library that has a bug causing data corruption. You can monkey-patch the problematic function to prevent the bug from manifesting while awaiting an official fix.
import problematic_library def patch_bug_function(): # Monkey-patch the problematic function problematic_library.buggy_function = fixed_function # Apply the patch patch_bug_function() |
Dynamic Behavior Alteration
Monkey-patching enables dynamic changes to the behavior of classes and modules at runtime. This can be particularly useful for adding or modifying functionality based on runtime conditions or user preferences.
Suppose you have a configuration module that reads settings from a file. You can use monkey-patching to override specific settings at runtime, allowing users to customize the application behavior without modifying the original configuration.
import config_module def customize_configuration(): config_module.application_mode = ‘custom’ # Apply the customization customize_configuration() |
Best Practices in Monkey Patching
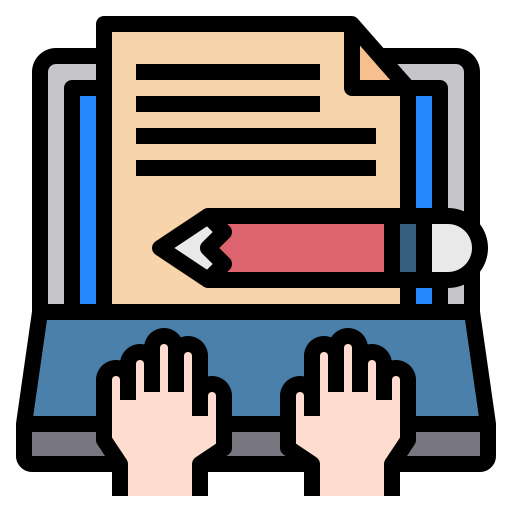
When it comes to Python monkey-patching, a set of best practices should be adhered to:
Documentation
Proper documentation is a cornerstone of responsible monkey patching. It helps developers understand why a patch was applied, what it modifies, and its intended behavior. Without clear documentation, future developers may struggle to grasp the purpose and impact of the patch.
Best Practices:
- Document the reason for the monkey patch: Explain why the patch is necessary, such as addressing a bug, adding new functionality, or adapting to changing requirements;
- Describe the affected code: Specify the classes, functions, or methods that are being patched;
- Include usage examples: Provide code snippets demonstrating how to apply and revert the patch.
Example Documentation:
# Monkey Patch Explanation: # This monkey patch is applied to fix a critical bug in the XYZ library. # It affects the `problematic_function` in the `xyz_module`. # Usage: Apply this patch using `apply_patch()` and revert it with `revert_patch()`. |
Scope Limitation
Monkey patches should have a limited scope to minimize the chances of conflicts, unintended consequences, and code maintainability issues. Limiting the scope helps isolate changes to specific areas of code.
Best Practices:
- Patch only what is necessary: Focus on modifying or extending the smallest possible part of the codebase to achieve the desired outcome;
- Avoid global changes: Refrain from patching core Python modules or widely used libraries unless there is no other viable solution.
Example of Scoped Monkey Patch:
# Good practice: Scoped monkey patching def custom_method(): # Define custom behavior pass # Apply the patch to a specific class or module original_method = library_module.problematic_method library_module.problematic_method = custom_method # Revert the patch when it’s no longer needed library_module.problematic_method = original_method |
Testing
Thorough testing is essential to ensure that monkey patches work as intended and do not introduce unexpected side effects. Without proper testing, patches may lead to subtle bugs that are challenging to diagnose.
Best Practices:
- Create comprehensive test cases: Develop test cases that cover various scenarios, including edge cases and corner cases;
- Automate testing: Implement automated tests that can be run regularly to catch regressions;
- Test in isolation: Isolate the patched code during testing to prevent interactions with other parts of the system.
Testing Example:
def test_custom_method(): # Ensure that the custom method behaves as expected assert custom_method() == expected_result def test_original_behavior(): # Verify that the original behavior is restored after patch removal assert library_module.problematic_method() == original_behavior |
Avoid Overuse
Monkey patching should be used sparingly and as a last resort. Overusing this technique can lead to code that is hard to understand, debug, and maintain.
Best Practices:
- Consider alternative solutions: Explore alternative approaches, such as subclassing or creating wrapper functions, before resorting to monkey patching;
- Assess long-term impact: Evaluate the long-term implications of applying a monkey patch, as it may lead to code that is difficult to maintain or upgrade;
- Review and refactor: Periodically review the codebase for unnecessary patches and refactor them if better solutions become available.
Conclusion
Monkey patching in Python is a powerful technique, offering the flexibility to modify code at runtime. However, with great power comes great responsibility. It’s essential to use monkey patching judiciously and follow best practices to minimize negative impacts on your codebase’s health and maintainability. Remember, a well-patched code can be a boon, but a recklessly patched one can be your worst nightmare.
FAQs
Generally, monkey patching should be used cautiously. It’s often seen as a last resort due to its potential to complicate code maintenance and readability.
Yes, if not done carefully. It can introduce bugs and unexpected behaviors, especially if the original codebase changes.
No, the concept exists in other languages too, but its implementation and use can vary.