Python’s programming language landscape saw a significant shift with the introduction of lambda functions. Commonly seen in languages like JavaScript, these anonymous functions were now part of Python’s syntax family. While they often take a backseat or are incorrectly utilized due to their presumed complexity, this article serves to shed ample light on the concept, its usage, and potential pitfalls.
Traditionally, a Python function could be defined through the def keyword:
def average(x, y):
return (x+y)/2
To calculate the average of two numbers, this function would be invoked as follows:
avg = average(2, 5)
In this instance, the value of avg would be 3.5. But Python allows us another way to define this average function using lambda syntax:
average = lambda x, y: (x+y)/2
Testing this lambda function yields the same outcome. However, the syntax differs significantly because the arguments x and y are defined without parentheses, and the return statement is inherently implied.
Despite the apparent simplicity, lambda functions come with their own set of unique attributes. They must be confined to a single line and lack docstrings for documenting the function’s purpose or usage. If you run help(average) on both definitions, you will encounter significantly different outputs.
From a functional standpoint, both definitions deliver identical results, making the difference between them rather nuanced. Lambda functions’ main boon lies in their anonymity—they don’t demand a name, and assigning a name, as demonstrated above, is generally seen as bad practice.
So, where can one leverage lambda functions over regular functions? Many tutorials focus on sorting a list with lambda functions. Let’s explore this, and more.
Consider the list below:
var = [1, 5, -2, 3, -7, 4]
To sort these values, you would use:
average = lambda x, y: (x+y)/2
But what if you want to sort the values based on their distance from a given number? This scenario calls for a function that calculates distance and sorts the values accordingly. The sorted function comes with a key= argument that can be used for this purpose:
def distance(x):
return abs(x-1)
sorted_var = sorted(var, key=distance)
# Output: [1, 3, -2, 4, 5, -7]
The same result can be achieved with a lambda function:
sorted_var = sorted(var, key=lambda x: abs(x-1))
Some might argue that lambda functions make the code more readable and compact, as the operation applied to each element is apparent without hunting for the function’s definition in the code.
Lambda functions also pair well with map, a Python function that applies a function to each item in a list:
def distance(x):
return abs(x-1)
sorted_var = sorted(var, key=distance)
# Output: [1, 3, -2, 4, 5, -7]
The same result can be produced with lambda functions:
sorted_var = sorted(var, key=lambda x: abs(x-1))
Again, it’s arguable that lambda functions offer better readability in this context. Additionally, lambda functions can prove handy when working with libraries like Pandas.
Leveraging Lambda Functions with the Qt Library
The power of lambda functions isn’t restricted to Python’s built-in features. They can be utilized in tandem with Python libraries such as Qt to enhance the functionality and workflow of your programs. If you are new to building user interfaces or unfamiliar with the Qt library, consider exploring our beginner’s guide to Qt programming. Here, we will delve into how to harness lambda functions within Qt, using a simple example.
Consider a basic PyQt application, which displays a button:
sorted_var = sorted(var, key=lambda x: abs(x-1))
Upon running this program, you will see an application window with a button labeled ‘Click Here!’. Nevertheless, clicking the button doesn’t initiate any actions. To introduce interactivity, we need to link an action to the button click event. In Qt, this action is defined as a function.
Suppose we want to output a message to the console every time the button is clicked. We can achieve this by adding the following line right before app.exit:
button.clicked.connect(lambda: print('Button Clicked!'))
With this addition, re-running the program will print ‘Button Clicked!’ to the console each time the button is pressed. This demonstrates how lambda functions can act as slots for signals, enhancing program interactivity and readability. In this context, readability indicates that developers can immediately understand what happens upon a button click, without the need to track down a separately defined function.
However, while lambda functions can streamline and simplify your code, they should be used judiciously. Lambda functions are restrained to a single line, which means they are not suitable for complex actions. Also, as anonymous functions, they lack docstrings which typically document a function’s purpose, usage, and return values.
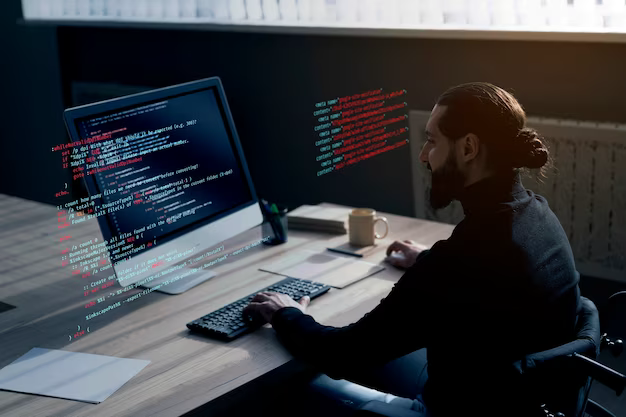
Practical Uses of Lambda Functions in Python
Python’s lambda functions, with their unique single-line constraint, are particularly well-suited for circumstances where the desired behavior can be achieved with uncomplicated syntax. Lambda functions offer a powerful yet concise way to encode logic without the overhead of defining a full multi-line function.
A typical scenario where lambda functions shine is as callable arguments for other functions. For instance, consider a data transformation operation on a pandas DataFrame using the apply() method. The apply() method expects a function as an argument to process the DataFrame’s data.
import pandas as pd
data = {'Name': ['John', 'Anna', 'Peter'],
'Age': [28, 24, 35],
'Country': ['USA', 'Germany', 'France']}
df = pd.DataFrame(data)
# Use a lambda function to transform 'Age' by adding 10 to each value
df['Age'] = df['Age'].apply(lambda x: x + 10)
In this example, the lambda function succinctly defines the transformation logic inline, enhancing code clarity and readability.
Another practical application of lambda functions is in the realm of user interface development using the Qt library. In Qt, actions triggered by user interactions (such as button clicks) are connected via signals to functions, or slots as they are known in Qt parlance.
from PyQt5.QtWidgets import QApplication, QPushButton
app = QApplication([])
button = QPushButton('Click Me!')
button.show()
# Use a lambda function as a slot to print a message when the button is clicked
button.clicked.connect(lambda: print('You clicked the button!'))
app.exit(app.exec())
Here, a lambda function is used as a slot to print a message whenever the user clicks the button. This application of lambda functions directly embeds the action within the signal connection, making the code more understandable.
Despite their versatility, it’s important to understand lambda functions’ limitations. If a solution requires complex logic or extensive multi-line code, a traditional function is a more apt choice. Functions that need to be reused also benefit from a traditional function definition.
The key to correctly using lambda functions lies in striking a balance: use them to foster readability and simplicity in your code, but don’t force their usage in situations where traditional functions would be more suitable.
Synchronization in Python
In the world of Python programming, synchronization plays a pivotal role in ensuring the smooth execution of concurrent processes. Synchronization mechanisms, such as locks, semaphores, and mutexes, enable developers to manage shared resources and prevent conflicts among threads or processes.
Lambda functions, while powerful and concise, are not a panacea for synchronization challenges. When dealing with multi-threaded or multi-process applications, it is essential to implement proper synchronization to avoid race conditions and data corruption.
For instance, if multiple threads or processes access and modify shared data concurrently, it can lead to unpredictable and erroneous outcomes. In such cases, synchronization tools like locks can be employed to ensure that only one thread or process accesses the shared data at any given time, preventing conflicts and maintaining data integrity.
Conclusion
Lambda functions, a feature of Python that adopts the philosophy of simplicity and readability, can dramatically streamline certain coding scenarios. By understanding the appropriate usage contexts and limitations of lambda functions, Python developers can harness their potential to write succinct, readable, and efficient code. Whether you’re transforming data in pandas or creating interactive user interfaces with Qt, lambda functions offer a powerful tool to enhance your Python programming prowess.