Python descriptors offer a powerful mechanism for managing class attribute access and modification. This sophisticated technique is especially prominent when properties in a class are manipulated through getter and setter methods, creating an illusion of accessing a single attribute. This article delves into the inner workings of the property decorator, providing insights into custom implementation strategies for effective attribute management. At the heart of Python’s approach to attribute management are descriptors. Descriptors are special types of objects that define how a specific attribute should be accessed and modified. The property decorator is a built-in Python feature that simplifies the creation of getters and setters, enabling controlled access to an attribute while keeping the interface simple and intuitive for the user.
One of the remarkable abilities of descriptors is their capacity to handle attribute access while also allowing these attributes to reference the class they belong to. This functionality is incredibly useful for scenarios where attributes of a certain type need to be registered within their parent class. Such a feature is invaluable in situations where class settings need to be dynamically altered, or where a class’s behavior depends on its attributes.
The article further explores how to define these self-aware attributes and manage potential inheritance issues that arise in complex class hierarchies. When a subclass inherits from a parent class with these descriptors, careful handling is needed to ensure that the subclass’s attributes are managed independently of the parent class. This ensures a clean and bug-free implementation, particularly in large and intricate object-oriented systems. Python’s descriptor system, exemplified by the property decorator, is a robust and elegant solution for attribute management. By understanding and utilizing these tools, developers can craft more flexible, maintainable, and efficient classes. The article aims to provide a comprehensive understanding of these concepts, empowering readers to implement custom solutions that leverage Python’s advanced object-oriented capabilities.
The Role of Properties in Python Descriptors
Embracing Python’s object-oriented nature often leads to the discovery of useful tools like the `@property` decorator, which provides a clean, readable, and efficient way to manage class attributes. The `@property` decorator is a built-in feature that transforms a method into a “getter” for a read-only attribute, allowing methods to be accessed like attributes. To illustrate, consider the following example:
```python
class MyClass:
_var = 0
@property
def var(self):
print('Getting Var')
return self._var
```
In this example, when the `var` method of `MyClass` is called, it behaves like a class attribute. Upon accessing `var`, the method under the `@property` decorator is executed, printing the statement “Getting Var” and then returning the value of `_var`. This demonstrates the decorator’s ability to transform a method’s behavior, embedding additional functionalities into what appears to be a simple attribute access. This transformation of methods into attribute-like properties using `@property` is further elaborated in the article. It’s a powerful aspect of Python, allowing for more controlled access to attributes, and can be extended to include setter and deleter functionalities as well, providing a full-fledged property management system.
It’s important to note that Python, unlike some other languages, lacks truly private attributes. Conventionally, a single underscore prefix (e.g., `_var`) is used to indicate that an attribute is intended for internal use within the class and should not be accessed or modified directly from outside its class. However, this is a convention rather than a strict enforcement by the Python language itself. Python’s philosophy trusts the developer’s discretion in respecting these conventions, emphasizing readability and simplicity over rigid access control. The use of the `@property` decorator and the underscore prefix for attribute naming are examples of how Python strikes a balance between flexibility and control in object-oriented programming. These tools and conventions empower developers to write clean, maintainable, and effective code while leveraging Python’s dynamic and expressive nature.
Beyond Basic Functionality: Advanced Uses of Properties
Properties in Python offer a unique way of controlling access to class attributes, blending the simplicity of attribute access with the control of getter and setter methods. This is particularly useful in complex programming scenarios, such as device control or data validation, where direct manipulation of class attributes might lead to errors or inconsistencies.
Consider the provided code example:
```python
class MyClass:
_var = 0
@property
def var(self):
print('Getting var')
return self._var
@var.setter
def var(self, value):
print('Setting var')
self._var = value
```
In this class, `MyClass`, the attribute `_var` is intended to be private (as suggested by the underscore prefix). Direct access to such private attributes is typically discouraged in object-oriented programming, as it can lead to a breach of the encapsulation principle. The `@property` decorator is used to define a method that acts as a getter for the attribute. When you access the attribute `var`, it automatically calls the `var` method, which returns the value of `_var`. This allows the class to control how the attribute is accessed. For instance, the class might want to log every access to the attribute, perform some computation, or fetch the value from an external source.
The `@var.setter` decorator is then used to define a method for setting the value of `_var`. Whenever you assign a value to `var`, this setter method is called, allowing the class to control or validate the value before it’s actually set. For example, the class might want to check that the value is within a certain range, convert it to a specific type, or update other dependent attributes.
This approach has several benefits:
- Encapsulation: It keeps the internal representation of the state hidden from the outside. This allows the internal implementation to be changed without affecting code that uses the class;
- Validation: When setting a value, the setter method can include checks and validations, ensuring the object always remains in a valid state;
- Abstraction: The user of the class doesn’t need to know how the values are retrieved or set. They can treat properties as simple attributes, even though complex logic might be running behind the scenes;
- Ease of Use: It allows for a more natural, attribute-like syntax while providing the full control of getter and setter methods;
- Flexibility: You can start with a simple public attribute and later change it to a property without modifying the interface of the class.
Properties are thus an elegant way to ensure that the principles of good object-oriented design are adhered to while also providing a clear and concise interface for class interaction. They are especially useful in scenarios where the underlying data may change dynamically or requires validation.
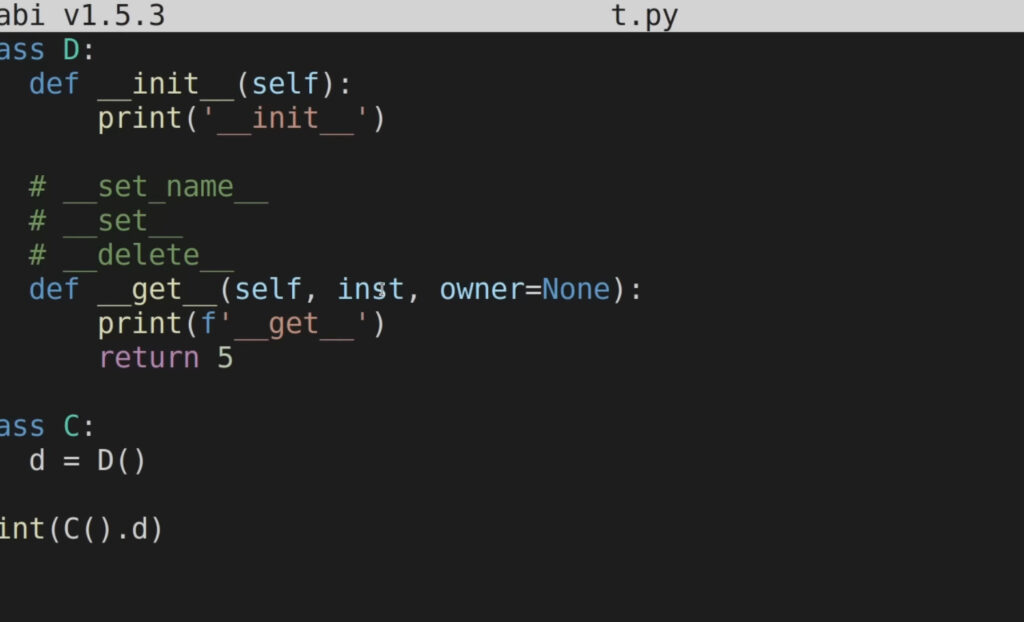
Expanding the Descriptor Toolbox
A descriptor is a class that implements any of the methods `__get__`, `__set__`, and `__delete__`. When an instance of such a class is assigned as a class attribute, Python automatically uses these methods to control access to the attribute. This mechanism provides a lower-level, more granular control over attribute behavior compared to the `@property` decorator.
Consider the following example of a custom descriptor class:
```python
class MyClass:
_var = 0
@property
def var(self):
print('Getting var')
return self._var
@var.setter
def var(self, value):
print('Setting var')
self._var = value
```
In this example, `MyDescriptor` implements `__get__` and `__set__` methods:
- `__get__` Method: This is invoked when the attribute is read. It can be used to return the value or compute it dynamically;
- `__set__` Method: This is called when the attribute is assigned a new value. Here, you can include validation logic, as shown in the example, where it checks if the assigned value is an integer.
Here are some advantages of using custom descriptors:
- Enhanced Validation: Descriptors allow for complex validation rules or type checking when attributes are assigned;
- Reusability: A single descriptor class can be reused across multiple attributes or even different classes;
- Encapsulation: They help in maintaining a clean separation between the attribute value and the logic for setting or getting the value;
- Complex Logic: Descriptors can implement intricate logic for attribute access, which goes beyond simple value setting and retrieval. For example, they can automatically update related attributes, log access, or interact with external resources;
- Consistency: They ensure that all instances of a class will use the same logic for accessing certain attributes, promoting consistency across the application.
In summary, while the `@property` decorator is suitable for straightforward scenarios, custom descriptors like `MyDescriptor` offer a more advanced and versatile approach for managing attribute access and encapsulation in Python. They are particularly useful when you need to enforce specific data types, maintain consistency, or implement complex attribute interaction patterns.
Developing Custom Descriptors
To further tailor attribute access in Python, one can develop custom descriptors. These are classes that define how attributes are accessed and modified. By implementing specialized `__get__` and `__set__` methods, you can control attribute access in a more granular way. This article will guide you through creating such descriptors and using them effectively.
Descriptors are powerful tools in Python. They allow you to define reusable properties that can be applied to different classes. When you define a descriptor, you essentially create a class that implements any of the following methods: `__get__`, `__set__`, and `__delete__`. Each of these methods corresponds to an aspect of managing an attribute: retrieving, setting, or deleting it. The `__get__` method is called to retrieve the attribute value. This method can be used to implement custom retrieval logic, such as type checking or computation. For example, you could use it to convert internal data into a user-friendly format. The `__set__` method is invoked when a value is assigned to the attribute. This is where you can add logic for validation or type conversion. By carefully defining this method, you can ensure that your attributes always have valid data.
You can also create read-only or write-only properties using descriptors. A read-only property is one that can be read but not modified. To create a read-only property, define a descriptor with a `__get__` method but no `__set__` method. Conversely, a write-only property can be modified but not read. This is less common but can be achieved by implementing `__set__` without `__get__`.
Custom descriptors in Python offer a flexible way to manage attribute access and modification. By defining `__get__` and `__set__` methods, you can control how attributes are retrieved and changed, and even implement read-only or write-only properties. This article has provided a basic overview of how to create and use custom descriptors effectively.
Advanced Descriptor Features and Considerations
The article delves into some of the advanced features of Python descriptors, highlighting their versatility and power. One such feature is using descriptors as decorators, a technique that can significantly enhance the functionality of class attributes. By leveraging the `__call__` method, descriptors can be designed to exhibit dynamic behavior when used, offering a level of control and flexibility that goes beyond traditional attribute access patterns.
The `__call__` method in Python allows an object to be called like a function. When applied in the context of descriptors, it opens up possibilities for custom validation, transformation, or other operations every time the attribute is accessed or modified. This method can be particularly useful for setting boundaries for acceptable attribute values. For instance, a descriptor can be designed to enforce type checks, value ranges, or other constraints, ensuring that the attribute values remain within specified limits.
Additionally, the article touches upon the `__set_name__` method introduced in Python 3.6, which offers new possibilities for manipulating descriptor owners more straightforwardly. The `__set_name__` method is called when a class is created, allowing the descriptor to know both the name of the attribute it is managing and the class to which it belongs. This feature simplifies the process of connecting descriptors with their owner classes, making it easier to handle scenarios involving inheritance and attribute management across different classes. This comprehensive exploration of Python descriptors and the property decorator is aimed at providing a deeper understanding of these powerful tools. The article highlights how these features can be effectively implemented to solve various programming challenges. Whether it’s enforcing data integrity, customizing attribute access, or managing class properties in sophisticated ways, descriptors and the property decorator offer Python programmers a rich set of capabilities. By equipping readers with this knowledge, the article aims to enable them to implement their own solutions more effectively, leveraging the full potential of Python’s object-oriented programming features.
Harnessing `__set_name__` for Attribute Registration in Python
In the realm of Python programming, there are scenarios where understanding the origin of an attribute within its owner class becomes crucial. This is particularly relevant in situations where handling specific types of attributes requires them to be registered in their defining class. Imagine a scenario involving a complex device with various settings. In such a case, it becomes important to identify and manage all these settings residing within the device efficiently. The key to achieving this is by using attributes capable of self-registration in the owning class, a concept that leverages the dynamic and introspective nature of Python.
Self-registering attributes can be implemented in Python through the use of descriptors or metaclasses. Descriptors are special class objects that define how access to an attribute is controlled. They are typically used to manage the attributes of another class by defining methods like `__get__`, `__set__`, and `__delete__`. By implementing a descriptor, you can create attributes that automatically register themselves within their owner class whenever they are declared. This is particularly useful for dynamically tracking attributes or settings in a complex system.
For example, consider a class representing a device, which may have various settings like volume, brightness, or mode. By using self-registering attributes, each time a new setting is added to the class, it can automatically be added to a registry within the class. This registry can then be used to introspect the device’s capabilities, iterate through its settings, or perform other meta-level operations.
Here’s a simplistic illustration:
```python
class SelfRegisteringAttribute:
def __set_name__(self, owner, name):
owner._registry.append(name)
class Device:
_registry = []
volume = SelfRegisteringAttribute()
brightness = SelfRegisteringAttribute()
# Other settings...
# Later, one can access the registry:
device = Device()
print(device._registry) # Output: ['volume', 'brightness']
```
In this example, the `SelfRegisteringAttribute` descriptor automatically adds the names of the attributes it manages to the `_registry` list of the `Device` class. This way, the `Device` class has an automatic record of all its settings.
Such techniques are particularly useful in scenarios where components or attributes need to be dynamically discovered, validated, or managed. It reflects Python’s capability to handle advanced programming patterns with relative ease, providing developers with tools to build sophisticated, introspective, and self-managing systems. This approach is not only elegant but also enhances the maintainability and scalability of the code, especially in complex software architectures where keeping track of numerous components is essential.
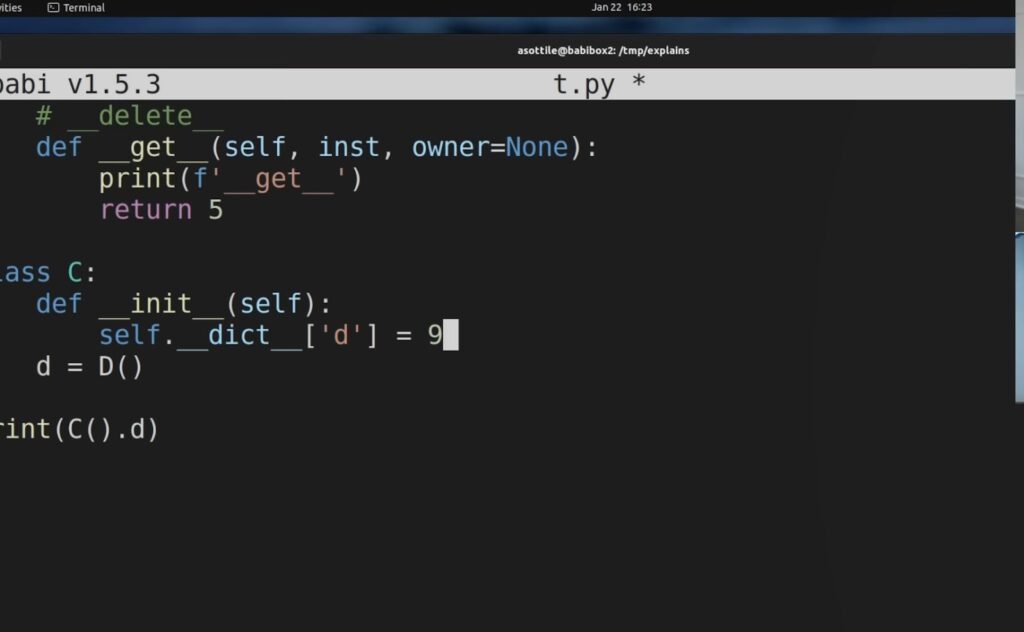
Implementing `__set_name__` for Self-Registration of Attributes
The journey begins with developing a descriptor leveraging the `__set_name__` method, which is quite similar to previous descriptor examples:
```python
class MyDescriptor:
def __init__(self, fget=None, fset=None):
# ...
def __set_name__(self, owner, name):
# ...
def __get__(self, instance, owner):
# ...
def __set__(self, instance, value):
# ...
def setter(self, fset):
# ...
```
Using this descriptor in a class like `MyClass`, one observes an intriguing behavior:
```python
class MyClass:
_var = 0
# ...
>>> from descriptors import MyClass
# ...
>>> my_class = MyClass()
>>> print(my_class._descriptors)
['var']
```
Before even creating an instance of `MyClass`, `__set_name__` assigns a name and links it to the owner class. This mechanism allows the storage of all `MyDescriptor` instances in a list, though one could also opt for a dictionary to include additional data like the latest value update or update timestamps.
Addressing Inheritance Issues in Descriptor Registration
While the approach is effective, it encounters a significant challenge with inheritance. For instance, if `MyOtherClass` inherits from `MyClass`, both classes end up sharing the same list of descriptors due to the mutable nature of Python lists. This results in shared data across parent and child classes, which may not always be desirable.
```python
class MyOtherClass(MyClass):
# ...
>>> my_class = MyClass()
>>> my_other_class = MyOtherClass()
>>> my_class._descriptors
['var', 'var1', 'new_var']
>>> my_other_class._descriptors
['var', 'var1', 'new_var']
```
The shared list of descriptors is a manifestation of how mutable data types work in Python. Addressing this issue is not straightforward, but innovative solutions do exist. One such solution, proposed by Hernán Grecco for the Lantz project, offers an elegant way to handle descriptor registration with inheritance, ensuring distinct descriptor lists for each class. This exploration into `__set_name__` and descriptor management highlights both the potential and complexities of attribute registration in Python, offering a glimpse into advanced techniques for handling class attributes and inheritance effectively.
Managing Inheritance and Subclassing Built-In Data Types in Python
In the intricate world of Python programming, one sometimes ventures into subclassing built-in data types. This practice, while somewhat esoteric, can be highly beneficial when executed with a clear purpose. Subclassing a standard data type like a list or a dictionary allows for the extension of their functionalities in a way that’s tailored to specific needs.
Let’s consider an example of subclassing a list:
```python
class MyList(list):
pass
var = MyList([1, 2, 3])
# Standard list operations...
```
In this example, `MyList` is a subclass of the built-in `list` class. This means it inherits all the methods and properties of a regular list, but it also allows for additional functionalities and attributes specific to `MyList`. You could, for instance, add methods to process data in a specific way that’s relevant to your application. One of the key advantages of subclassing is that it enables the addition of unique attributes to the new class. For instance, in the `MyList` class, you could include methods that are not present in the standard list class. This could be something as simple as adding a method to calculate the average of the numbers in the list, or more complex operations tailored to your specific use case.
However, caution is advised when subclassing built-in types. One of the main concerns is the potential of overriding inherent methods of the list class. If you override a method unintentionally, it could change the fundamental behavior of the list, leading to unexpected results. Therefore, it’s crucial to have a good understanding of the methods and properties of the built-in type you are subclassing. Another point to consider is the performance implications. While subclassing can provide powerful customization, it may come with a cost in terms of performance, especially if the subclassed methods are computationally intensive or if they are not as optimized as the built-in methods.
Additionally, subclassing built-in types can sometimes lead to confusing code, especially for other developers who might expect the standard behavior of these types. Therefore, it’s important to document any changes or additions thoroughly. In conclusion, subclassing built-in data types in Python can be a powerful tool for extending the functionality of these types and tailoring them to specific needs. However, it should be done with care and consideration, keeping in mind the potential impacts on performance, maintainability, and code clarity. By using subclassing judiciously and with a clear purpose, you can harness the power of Python’s flexibility to create more efficient and effective code.
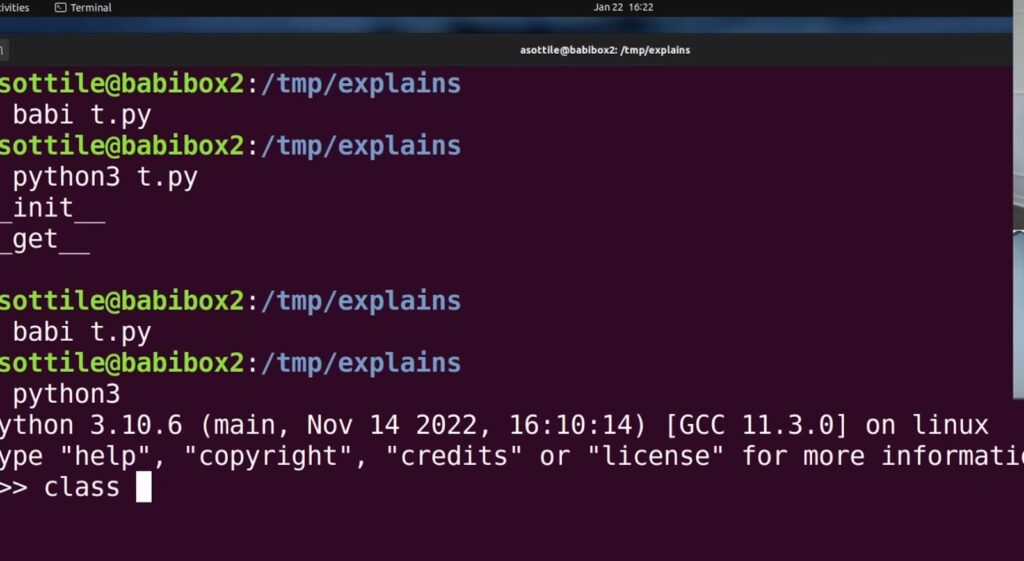
Checking Ownership in Descriptor Implementation
To prevent subclasses from inadvertently affecting their parent classes in Python, especially when working with descriptors, a method involving the registration of the owner class within the descriptor list itself is employed. This strategy hinges on the creation of a custom list for descriptor registration and a careful management of ownership within the descriptor itself.
Consider the scenario where we have a custom list class:
```python
class MyList(list):
pass
```
This class serves as a base for our custom descriptors. The next step involves modifying the `MyDescriptor` class, particularly the `__set_name__` method, to manage ownership of descriptors in a way that respects class hierarchies:
```python
class MyDescriptor:
def __set_name__(self, owner, name):
# Implementation...
```
In this method, the ownership of the descriptor is carefully managed. The process involves several steps:
- Check for Existing Descriptors: The method first checks if there is an existing `_descriptors` attribute in the owner (the class where the descriptor is being used). This attribute is intended to store a list of descriptors belonging to the class;
- Verify Ownership: If `_descriptors` already exists, the method needs to verify whether it belongs to the current class (`owner`) or a parent class. This is done by comparing the `owner_class` attribute of the descriptor list with the qualified name (`__qualname__`) of the owner class;
- Handle Inheritance: If the `owner_class` differs from the `__qualname__` of the owner, this indicates that the class is a subclass inheriting the descriptor. In such a case, a new `_descriptors` attribute is created for the subclass. This ensures that the subclass has its own independent list of descriptors, preventing any inadvertent effects on the parent class;
- Append Descriptor: Finally, the current descriptor’s name is appended to the appropriate list (`_descriptors`), either in the current class or the subclass, depending on the inheritance check.
This approach is particularly useful when dealing with complex class hierarchies involving multiple levels of inheritance. By ensuring that each class has its own list of descriptors, we avoid scenarios where changes in a subclass could unintentionally affect parent classes. This method of managing descriptors is an example of Python’s flexibility in handling advanced object-oriented programming concepts, allowing for more robust, maintainable, and error-resistant code. It reflects a deep understanding of Python’s class model and the descriptor protocol, showcasing how Python’s capabilities can be utilized to create sophisticated and well-structured object-oriented designs.
Inheritance Working as Intended
With this approach, where a subclass extends the functionality of its parent class, inheritance in Python functions correctly from parent to child. This can be demonstrated by examining specific attributes or methods in both the parent and child classes. The seamless inheritance of properties and methods is one of the cornerstones of object-oriented programming in Python, allowing for code reuse and extension. In the context of Python classes, let’s consider an example where `MyClass` is a parent class, and `MyOtherClass` is a subclass of `MyClass`. By using inheritance, `MyOtherClass` inherits all the attributes and methods of `MyClass`, unless explicitly overridden. This can be illustrated through an example:
```python
class MyClass:
# Definition of MyClass with its methods and properties
class MyOtherClass(MyClass):
# MyOtherClass extends MyClass
pass
my_class = MyClass()
my_other_class = MyOtherClass()
# ...
```
In this setup, `MyOtherClass` inherits all the features of `MyClass`. This includes any descriptors defined in `MyClass`. Descriptors in Python are a way to create managed attributes in classes. They include methods like `__get__`, `__set__`, and `__delete__` to control attribute access. If `MyClass` contains such descriptors, `MyOtherClass` will inherit them. Examining the `_descriptors` attribute (if it exists) in both classes would show how `MyOtherClass` inherits from `MyClass`. The `_descriptors` attribute is a Python convention for storing descriptor objects in a class. By inspecting this attribute, one can understand how attributes are being managed in both the parent and child classes.
Here’s how you might inspect these attributes:
```python
print(my_class._descriptors) # Shows descriptors of MyClass
print(my_other_class._descriptors) # Shows descriptors of MyOtherClass, inherited from MyClass
```
This inheritance model in Python is powerful and flexible, allowing developers to build complex hierarchies of classes that extend and modify behavior in a controlled manner. It’s important to understand how inheritance works in Python to design effective class structures. When subclassing, especially from standard library classes or complex custom classes, it’s crucial to be aware of how inherited methods and attributes interact with new ones defined in the subclass. This understanding helps in maintaining clear, functional, and bug-free code.
Concluding Thoughts on Descriptors
While this article has primarily focused on using descriptors akin to the `@property` decorator in Python, it’s important to understand that the versatility of descriptors extends far beyond this common usage pattern. Unlike the `@property` decorator, which is a convenient way to create a read-only attribute, general descriptors can be direct class attributes and offer profound control over attribute manipulation within a class. This is evident in Python’s official documentation, which provides detailed insights into the power and flexibility of descriptors.
Descriptors in Python are implemented using the descriptor protocol, which consists of methods like `__get__`, `__set__`, and `__delete__`. These methods enable the descriptor to control how attributes in a class are accessed, modified, or deleted. What makes descriptors particularly powerful is their ability to manage attributes not only in the instance of a class but also in the class itself. One of the key aspects of the descriptor protocol is its utility in specific tasks such as attribute registration within a class. This capability is especially useful in scenarios where access to the owner class is required during attribute definition. Descriptors provide a means to interact with the class state or properties in a way that regular methods or attributes can’t.
The adaptability of descriptors allows for advanced implementations. For example, they can be used for caching values to improve performance, setting timeouts for certain operations, or even for more complex patterns like lazy property evaluation. This demonstrates the utility of the descriptor protocol in complex Python programming scenarios. While descriptors are not a necessity in every programming scenario, their importance becomes apparent in situations that require a high level of control over how attributes are handled. This includes cases where attributes need to be computed on the fly, validated rigorously, or when their access needs to be restricted or monitored. Understanding and utilizing the full capabilities of descriptors can lead to more efficient, maintainable, and robust Python code, particularly in large-scale or complex applications.