Programming in Python often involves handling lists and tuples, seemingly similar yet pivotal in their underlying behavior. The distinction between mutable and immutable data types holds the key to robust programming. This article delves into their differences, implications, and optimal usage.
Lists and Tuples in Python
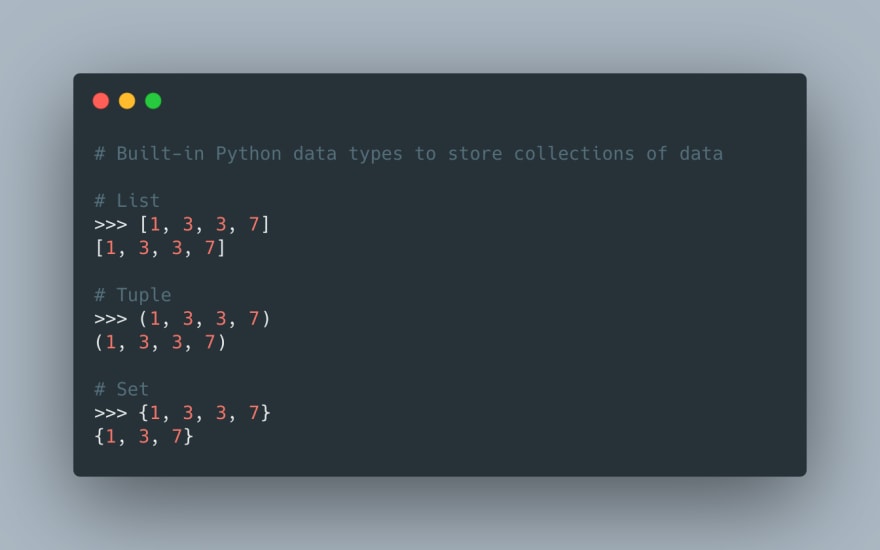
Python novices encounter lists and tuples, seemingly interchangeable yet fundamentally different. Understanding these distinctions between mutable and immutable data types is crucial for seamless programming. Lists, mutable entities defined with [], allow alterations of elements after creation. Conversely, tuples, defined with (), are immutable, forbidding any changes once defined.
The core differences extend beyond lists and tuples, encompassing various data types like integers, floats, strings, and more. Mutable types like lists, dictionaries, and sets permit in-place modifications, while immutable types ensure stability once assigned:
- Lists and tuples, fundamental data structures in Python, often perplex programmers due to their subtle differences and profound impact on code efficiency. While superficially similar, these entities serve distinct purposes;
- Lists, defined by square brackets [], are mutable sequences allowing modifications after creation. Contrastingly, tuples, enclosed in parentheses (), maintain immutability post-definition. This inherent difference dictates their application contexts;
- The choice between lists and tuples depends on use cases. Lists offer flexibility, enabling alterations, ideal for dynamic data. On the other hand, tuples’ immutability guarantees data integrity, ensuring safety for unchangeable elements;
- Understanding their performance characteristics is crucial. Tuples, being immutable, excel in faster access times, while lists demonstrate greater memory efficiency for expansion;
- Moreover, Python’s lists and tuples embody deeper nuances in handling mutable and immutable data types. They serve as entry points to comprehend Python’s broader data type landscape, encompassing integers, strings, sets, dictionaries, and more.
Delving into Python’s lists and tuples is a gateway to mastering data structures, facilitating efficient coding practices and optimal resource utilization.
Here’s a simple demonstration of lists and tuples in Python:
Lists:
# Creating a list
my_list = [1, 2, 3, 4, 5]
# Accessing elements in a list
print(“First element of the list:”, my_list[0]) # Output: 1
print(“Last element of the list:”, my_list[-1]) # Output: 5
# Modifying elements in a list
my_list[2] = 10
print(“Modified list:”, my_list) # Output: [1, 2, 10, 4, 5]
# Adding elements to a list
my_list.append(6)
print(“List after appending an element:”, my_list) # Output: [1, 2, 10, 4, 5, 6]
# Removing elements from a list
my_list.remove(4)
print(“List after removing an element:”, my_list) # Output: [1, 2, 10, 5, 6]
Tuples:
# Creating a tuple
my_tuple = (1, 2, 3, 4, 5)
# Accessing elements in a tuple
print(“First element of the tuple:”, my_tuple[0]) # Output: 1
print(“Last element of the tuple:”, my_tuple[-1]) # Output: 5
# Trying to modify a tuple (results in an error)
# my_tuple[2] = 10 # This line will raise a TypeError
# Combining tuples
new_tuple = my_tuple + (6, 7)
print(“Combined tuple:”, new_tuple) # Output: (1, 2, 3, 4, 5, 6, 7)
# Tuple unpacking
a, b, *rest = new_tuple
print(“Unpacked variables:”, a, b, rest) # Output: 1 2 [3, 4, 5, 6, 7]
This code snippet showcases basic operations with lists and tuples in Python, illustrating their characteristics such as mutability and immutability.
Mutable and Immutable Data Types
In Python, data types come in two flavors: mutable and immutable. Understanding the difference between them is crucial for writing efficient and reliable code. Here’s a breakdown:
Mutable Data Types:
- Can be changed after creation. You can modify their content, add or remove elements, essentially altering their internal structure;
- Examples: lists, dictionaries, sets;
- Operations like append, remove, or update directly modify the original object;
- Useful for: building and manipulating dynamic structures like shopping carts, user profiles, or game states.
Immutable Data Types:
- Cannot be changed after creation. Their content is fixed once created. Any attempt to modify them results in a new object with the desired changes;
- Examples: integers, floats, strings, tuples;
- Operations like adding or removing elements create new objects, leaving the originals untouched;
- Useful for: representing data that should remain consistent, like calculations, configuration values, or filenames.
Here’s a table summarizing the key differences:
Feature | Mutable Data Types | Immutable Data Types |
---|---|---|
Modify after creation | Yes | No |
Internal structure changes | Yes | No |
Operations modify original | Yes | No |
Examples | lists, dictionaries, sets | integers, floats, strings, tuples |
Use cases | Dynamic structures, data manipulation | Consistent data, configuration values |
Choosing the Right Data Type:
- Use mutable data types when you need to dynamically modify data structures;
- Use immutable data types when you need data to remain consistent and predictable, or when sharing data between threads requires immutability;
- Remember: immutable data types are generally faster to access and compare, while mutable data types offer more flexibility in data manipulation.
Benefits of Understanding Mutable vs. Immutable:
- Improved code clarity: Choosing the right data type makes your code more readable and maintainable;
- Reduced errors: Immutable data helps prevent accidental data corruption;
- Enhanced performance: Immutable data can be shared efficiently between threads, leading to faster execution.
Further Exploration:
- Check out Python documentation for detailed information on specific data types: https://docs.python.org/;
- Explore resources like Real Python’s guide on mutable vs. immutable types: https://realpython.com/lessons/immutable-vs-mutable/
Here’s an illustrative example showcasing the differences between mutable and immutable data types in Python:
Immutable Data Types:
python
# Immutable: integers x = 5 y = x # y references the same value as x x += 1 # Attempt to change x print("Immutable - Integer:") print("x:", x) # Output: 6 print("y:", y) # Output: 5 (y remains unchanged)
Mutable Data Types:
python
# Mutable: lists list_a = [1, 2, 3] list_b = list_a # list_b references the same list as list_a list_a.append(4) # Mutating list_a print("\nMutable - List:") print("list_a:", list_a) # Output: [1, 2, 3, 4] print("list_b:", list_b) # Output: [1, 2, 3, 4] (list_b changes as it refers to the same list
Explanation:
- Immutable data types, like integers, don’t change in place. When you perform operations on them, they create new objects;
- Mutable data types, such as lists, can be modified in place. Changes to a mutable object affect all references pointing to it.
Understanding these distinctions between mutable and immutable data types is crucial for effective programming in Python, aiding in predictable behavior and preventing unexpected side effects in code.
Mutable Objects in Functions
When working with mutable objects in functions, it’s important to understand how Python handles references and object identity. Here’s a breakdown:
Passing Mutable Objects:
- When you pass a mutable object (e.g., list, dictionary) to a function, you’re actually passing a reference to that object, not a copy. This means both the function and the original caller share the same object in memory;
- Any modifications made to the object inside the function will also be reflected in the original object outside the function.
Example:
Python
my_list = [1, 2, 3]
def modify_list(data):
data.append(4)
modify_list(my_list)
print(my_list) # Output: [1, 2, 3, 4]
Avoiding Unintended Modifications:
- If you want to avoid modifying the original object, you can:
- Pass a copy of the mutable object to the function. You can use methods like list.copy() or slicing to achieve this;
- Create a new mutable object inside the function and return it. This way, the original object remains untouched.
Example:
Python
my_list = [1, 2, 3]
def modify_list_copy(data):
new_data = data.copy()
new_data.append(4)
return new_data
modified_list = modify_list_copy(my_list)
print(my_list) # Output: [1, 2, 3]
print(modified_list) # Output: [1, 2, 3, 4]
Key Points:
- Passing mutable objects by reference can be convenient, but it can also lead to unexpected modifications if not handled carefully;
- Always be aware of the reference behavior when working with mutable objects in functions.
Choose the approach that best suits your desired outcome: modifying the original object or creating a new one.
Here’s an example demonstrating mutable objects within functions in Python:
Mutable Objects in Functions:
python
Copy code
def modify_list(input_list): # Modifying the list passed as an argument input_list.append(4) input_list[0] = 100 my_list = [1, 2, 3] print("Original list:", my_list) # Output: [1, 2, 3] modify_list(my_list) print("Modified list:", my_list) # Output: [100, 2, 3, 4]
Explanation:
- The function modify_list takes a list as an argument;
- Inside the function, the list is modified by appending an element and changing the value at index 0;
- When the function is called with my_list, the original list is modified within the function, showcasing how mutable objects can be altered in place.
This demonstrates how mutable objects, like lists, can be modified within functions, affecting the original object passed as an argument. It’s important to be mindful of this behavior, especially when dealing with mutable objects as function parameters to avoid unintended modifications.
Default Arguments in Functions
Default arguments are a powerful tool in Python functions, allowing you to:
- Simplify function calls: By providing pre-defined values for some arguments, you can avoid explicitly specifying them every time you call the function. This can make your code more concise and readable;
- Increase flexibility: Default arguments allow you to create functions that can handle various cases with minimal code changes. You can define different functionalities based on the provided arguments or their absence;
- Improve error handling: By setting sensible defaults, you can avoid potential errors caused by missing arguments. This can make your code more robust and user-friendly.
Here’s a breakdown of how default arguments work:
- Defining default arguments: You assign values to function parameters within the function definition itself. These values will be used if the corresponding argument is not provided when calling the function;
- Syntax: You use an = sign after the parameter to specify the default value. For example, def my_function(x, y=5):. Here, y has a default value of 5;
- Calling the function: You can call the function with or without the argument with a default value. If you omit it, the default value will be used.
Example:
Python
def greet(name="World", message="Hello"):
"""
Greets a person with a message.
Args:
name: The name of the person to greet (default: "World").
message: The message to greet with (default: "Hello").
Returns:
A string containing the greeting message.
"""
return f"{message}, {name}!"
print(greet()) # Output: Hello, World!
print(greet(name="Alice")) # Output: Hello, Alice!
print(greet(message="Good morning")) # Output: Good morning, World!
print(greet(name="Bob", message="How are you?")) # Output: How are you?, Bob!
Key Points:
- Default arguments are specified in the function definition;
- You can provide default values for any number of arguments;
- Calling the function with a missing argument uses the corresponding default value.
Default arguments can significantly improve the readability, flexibility, and robustness of your Python functions.
Your Own Immutable Objects
Creating immutable objects in Python involves redefining certain methods to enforce immutability. Here’s an example of implementing a custom immutable class:
Custom Immutable Class:
python
class MyImmutable: def __init__(self, var1, var2): self._var1 = var1 self._var2 = var2 @property def var1(self): return self._var1 @property def var2(self): return self._var2 def __setattr__(self, key, value): raise AttributeError("Can't modify immutable object") def __delattr__(self, item): raise AttributeError("Can't delete attributes in immutable object") # Creating an instance of the immutable class immutable_obj = MyImmutable(1, 2) # Accessing attributes (variables) print("Variable 1:", immutable_obj.var1) # Output: 1 print("Variable 2:", immutable_obj.var2) # Output: 2 # Trying to modify attributes (will raise an error) # immutable_obj.var1 = 10 # This line will raise an AttributeError
Explanation:
- The MyImmutable class has two private variables _var1 and _var2;
- Properties var1 and var2 are defined to access these private variables;
- The __setattr__ and __delattr__ methods are redefined to prevent modification and deletion of attributes, enforcing immutability;
- Attempts to modify or delete attributes of instances created from this class will raise AttributeErrors, maintaining object immutability.
This demonstrates a basic implementation of an immutable class in Python, showcasing how to prevent attribute modifications to ensure immutability.
Conclusion
Awareness of mutable and immutable types often goes unnoticed until obscure bugs surface in complex projects. Understanding their implications and employing proper strategies is fundamental to crafting robust and bug-free Python applications. Avoiding unexpected behaviors stemming from mutable types can prevent hard-to-track bugs, ensuring smoother programming experiences.