The essence of web frameworks lies in their ability to map URLs to actions, a fundamental process known as routing. While often overlooked, routing serves as the backbone of web applications, dictating user interactions and data access. In this comprehensive guide, we delve into the often underestimated artistry of routing within Flask, exploring its depth and potential for creating dynamic and scalable applications.
Flask Routes: Mapping Paths to Views
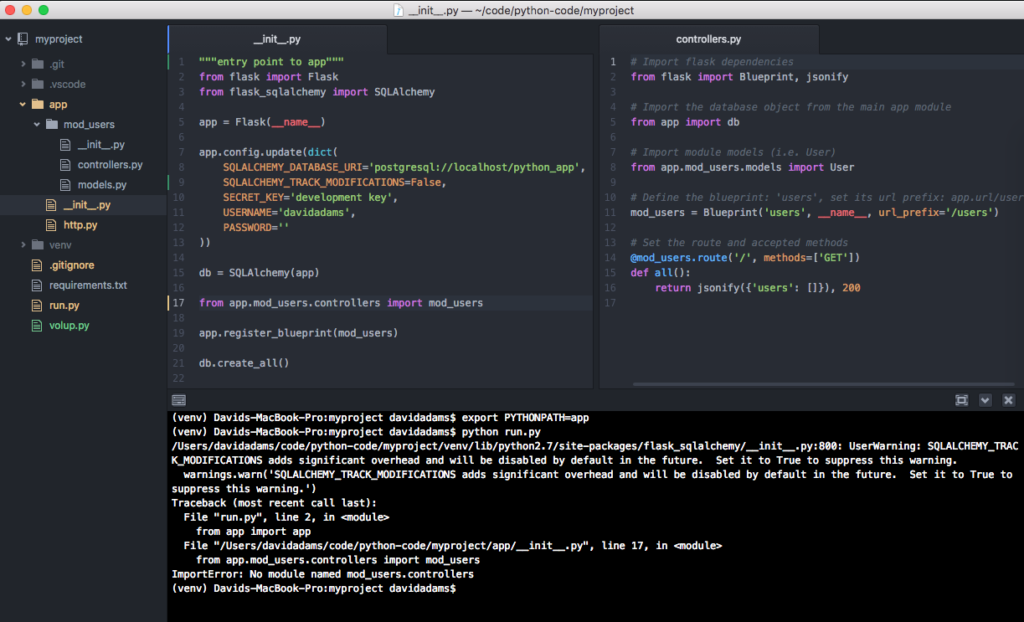
Flask routes are the URL patterns that the application uses to map requests to the appropriate view functions. They are defined using the @app.route() decorator, where @app refers to your Flask application instance.
Here’s an example:
python
from flask import Flask app = Flask(__name__) @app.route(‘/’) # This decorator maps the URL ‘/’ to the index function def index(): return ‘Welcome to the homepage!’ @app.route(‘/about’) # This decorator maps the URL ‘/about’ to the about function def about(): return ‘This is the about page.’ # Dynamic route that accepts a parameter @app.route(‘/user/<username>’) # This decorator maps URLs like ‘/user/some_username’ to the user_profile function def user_profile(username): return f’Profile page of {username}’ if __name__ == ‘__main__’: app.run(debug=True)
In this example:
- @app.route(‘/’) maps the root URL (‘/’) to the index() function, which returns a welcome message;
- @app.route(‘/about’) maps the URL ‘/about’ to the about() function, which displays information about the application;
- @app.route(‘/user/<username>’) defines a dynamic route that matches URLs like /user/some_username. The username variable in <username> is passed as an argument to the user_profile() function.
Routes can have dynamic components enclosed in < >, which can capture variable parts of the URL. These variables are then passed as arguments to the corresponding view function.
Remember, Flask relies on these routes to determine which view function should handle a specific URL request.
Route HTTP Methods
In Flask, the methods parameter in the @app.route() decorator allows you to specify which HTTP methods are accepted for a particular route. This is incredibly useful, especially when you’re building RESTful APIs and need to restrict access or perform different actions based on the HTTP method used.
In your example:
python
from flask import Flask app = Flask(__name__) @app.route(“/api/v1/users/”, methods=[‘GET’, ‘POST’, ‘PUT’]) def users(): # Logic goes here # This route ‘/api/v1/users/’ accepts GET, POST, and PUT requests if request.method == ‘GET’: # Logic for handling GET request pass elif request.method == ‘POST’: # Logic for handling POST request pass elif request.method == ‘PUT’: # Logic for handling PUT request pass
Here’s a breakdown of how this works:
- @app.route(“/api/v1/users/”, methods=[‘GET’, ‘POST’, ‘PUT’]) decorates the users() function to accept GET, POST, and PUT requests;
- Inside the users() function, you can use conditional statements (if, elif, else) based on request.method to execute specific logic depending on the type of request received.
This setup allows you to handle different HTTP methods (GET, POST, PUT, DELETE, etc.) within the same endpoint or route, executing specific logic based on the method used in the request.
Remember to import request from Flask (from flask import request) to access the request object that contains information about the incoming request, including the HTTP method.
Dynamic Routes & Variable Rules
Flask’s variable rules, denoted by the angled brackets (<variable_name>), allow you to create dynamic routes that can handle variable values in the URL. This feature is incredibly useful for creating routes that adapt to different user inputs or data in your application.
In your provided code snippets:
python
@app.route(‘/user/<username>’) def profile(username): # Logic for user profile page based on the provided username # Here, ‘username’ will be the variable extracted from the URL # Fetch user data, render a profile page, etc. pass @app.route(‘/<int:year>/<int:month>/<title>’) def article(year, month, title): # Logic for displaying an article based on the provided year, month, and title # ‘year’, ‘month’, and ‘title’ will be variables extracted from the URL # Fetch article data based on these variables, render the article, etc. pass
In the profile() function:
- The route /user/<username> expects a username in the URL. Whatever value is provided in place of <username> will be passed as an argument to the profile() function. You can then use this username to retrieve user-specific data or render a profile page.
In the article() function:
- The route /<int:year>/<int:month>/<title> expects an integer year, an integer month, and a title in the URL. It demonstrates how to handle multiple dynamic parameters in a single route. The provided values for year, month, and title will be passed as arguments to the article() function. You can use these values to fetch an article based on the publication date and title.
These dynamic routes enable you to create more flexible applications, allowing users to access specific content or functionalities based on the variable parameters in the URL.
Types of View Responses
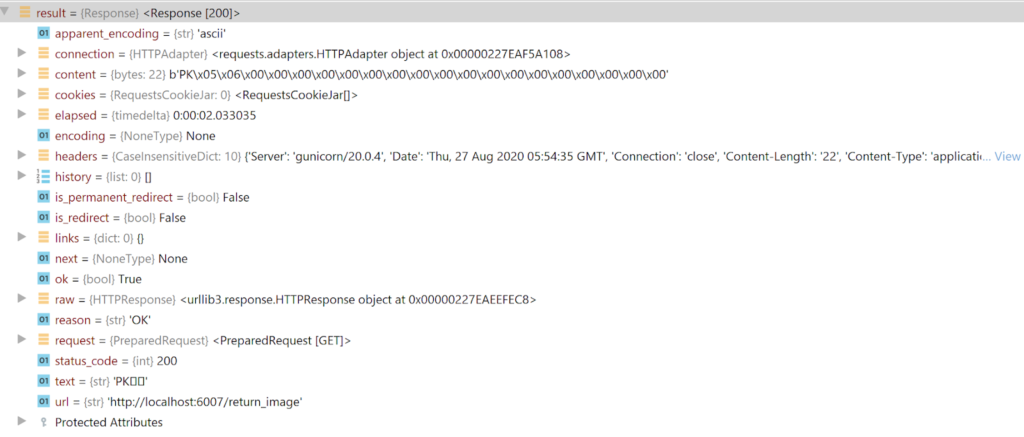
Flask views, the functions that handle requests in your application, can return various types of responses to suit different needs. Here’s a breakdown of the most common ones:
Rendering Page Templates
Flask routes often conclude by rendering page templates, providing responses, or redirecting users. The render_template() function in Flask is used to generate HTML pages using Jinja2 templates. To make this work, ensure your app is set to recognize a templates directory:
python
Copy code
from flask import Flask, render_template app = Flask(__name__, template_folder="templates")
The render_template() function is used within a route to serve an HTML page. For instance:
python
@app.route("/") def home(): """Serve homepage template.""" return render_template("index.html")
You can pass values to your templates as keyword arguments:
python
@app.route("/") def home(): """Serve homepage template.""" return render_template( 'index.html', title='Flask-Login Tutorial.', body="You are now logged in!" )
Making a Response Object
When endpoints need to respond programmatically rather than with HTML pages, make_response() is utilized. This function allows setting status codes and headers. It’s commonly used to provide information in the form of JSON objects:
python
from flask import Flask, make_response app = Flask(__name__) @app.route("/api/v2/test_response") def users(): headers = {"Content-Type": "application/json"} return make_response( 'Test worked!', 200, headers=headers )
Redirecting Users Between Views
The redirect() function is used to redirect users. It accepts a string representing the path to redirect the user to:
python
from flask import Flask, redirect app = Flask(__name__) @app.route("/login") def login(): return redirect('/dashboard.html')
Instead of directly providing URLs, it’s considered best practice to use url_for() which takes the view function’s name as input and outputs the URL route:
python
from flask import Flask, redirect, url_for app = Flask(__name__) @app.route("/login") def login(): return redirect(url_for('dashboard'))
Flask offers various response mechanisms like rendering templates, generating response objects, and redirecting users, each serving different purposes based on the needs of your application. These capabilities empower the creation of dynamic and interactive web applications.
Building Smarter Views
Developing strong routes involves mastering both the conceptual (soft) aspects, like adhering to MVC principles, and the practical (hard) aspects, such as using the available tools effectively.
Understanding MVC Principles
In Flask, following Model-View-Controller (MVC) principles is vital. Views should primarily handle response logic, not extensive business logic, keeping them lean and focused. This is a skill that develops through practice and learning from examples.
Essential Tools for Building Routes
The Request Object
The request object is a global object available in every route, encapsulating the request’s context. Here are some key properties of the request object:
- request.method: Identifies the HTTP method used (GET, POST, etc.). This helps create routes that respond differently based on the request method;
- request.args: Holds query-string parameters from the request;
- request.data: Contains the body of an object posted to a route;
- request.form: Provides access to form data submitted to the route;
- request.headers: Carries the HTTP response headers of the request.
Here’s an intricate example utilizing request properties in a single route from Flask-Login:
python
@app.route('/signup', methods=['GET', 'POST']) def signup_page(): signup_form = SignupForm(request.form) if request.method == 'POST': # Handling form submission if signup_form.validate(): # Extracting form fields name = request.form.get('name') email = request.form.get('email') # ...other form fields # Database operations, user creation, etc. # Rendering the signup page return render_template( '/signup.html', title='Create an Account | Flask-Login Tutorial.', form=SignupForm(), template='signup-page', body="Sign up for a user account." )
The “g” Object
The g object in Flask is for storing data that’s not part of the request object. It behaves somewhat like a global variable within the application context. Values can be assigned and accessed using g. However, excessive use of g might lead to confusion. Here’s a simple example:
python
from flask import g def get_test_value(): if ‘test_value’ not in g: g.test_value = ‘This is a value’ return g.test_value # To remove a value from g: @app.teardown_testvalue def remove_test_value(): test_value = g.pop(‘test_value’, None)
Balancing Skills for Efficient Routing
Mastering Flask routes requires a blend of understanding MVC architecture and utilizing Flask’s tools like the request and g objects. While the request object offers contextual data from incoming requests, the g object serves as a contextual store for values across a single request context. Balancing both ensures efficient and structured routing within your Flask application.
Additional Route-related Logic
Flask’s arsenal of decorators beyond @app.route() expands the capabilities of routes and allows handling requests and errors more effectively:
Additional Route Logic:
- @app.before_request(): Executes a function before every request. Useful for pre-processing tasks like user tracking, permission handling, or preserving session states;
- @app.endpoint(‘function_name’): Specifies an endpoint, an alternative way to map URLs to logic, often employed in larger applications involving Blueprints.
Error Handling
Flask simplifies error handling through @app.errorhandler():
python
@app.errorhandler(404) def not_found(): """Page not found.""" return make_response(render_template("404.html"), 404)
Here, @app.errorhandler(404) deals with 404 errors by rendering a custom template. Similar decorators can handle various HTTP error codes like 400 or 500, ensuring users receive appropriate responses.
Advanced Route Decorators via Plugins
Flask’s plugins offer powerful decorators for specialized functionalities:
- @login_required (from Flask-Login): Safeguards routes, allowing access only to logged-in users;
- @expose (from Flask-Admin): Creates views for a custom admin panel;
- @cache.cached() (from Flask-Cache): Caches routes for a specified duration (@cache.cached(timeout=50)).
Flask’s route capabilities exceed the basics, empowering developers to handle diverse scenarios. While newcomers start with fundamental route concepts, Flask’s flexibility enables immediate software development with the ability to add complexity incrementally.
With this diverse knowledge, you’re well-prepared to craft impressive applications. Now, it’s time to apply this knowledge and dive into coding adventures!
What does app Flask (__ name __) mean?
In Flask, Flask(__name__) is the creation of a Flask application instance. Let’s break it down:
- Flask: This is the class provided by the Flask framework that you’re importing to create your web application;
- (__name__): This is a Python predefined variable that represents the current module’s name. When you’re running your Python script directly (not importing it from another script), __name__ is set to ‘__main__’. However, if your script is imported as a module into another script, __name__ is set to the name of your script/module.
When you pass __name__ to Flask(), it helps Flask to determine the root path of the application, enabling Flask to locate important files and folders such as templates, static files, and more.
For instance, when Flask initializes, it uses this information to locate templates folder (if specified using template_folder) or static files (if specified using static_folder). This helps Flask to organize and serve web-related assets correctly based on their relative paths.
Here’s an example:
python
from flask import Flask app = Flask(__name__)
This line of code initializes a Flask application named app using the current module’s name (__name__) to set up the necessary paths and configurations for your Flask application.
How do you handle variables in a route in Flask?
In Flask, handling variables within routes is achieved using dynamic routes defined with variable rules. Variable rules are represented within the route URL using angled brackets (<variable_name>), allowing for dynamic URL generation and handling of varying inputs.
Here’s an example of handling variables in Flask routes:
python
from flask import Flask app = Flask(__name__) # Handling a username variable in the route @app.route('/user/<username>') def profile(username): return f'Profile page of {username}' # Handling multiple variables in the route @app.route('/post/<int:post_id>') def show_post(post_id): return f'Post ID: {post_id}' # Handling different variable types in the route @app.route('/path/<path:subpath>') def show_subpath(subpath): return f'Subpath: {subpath}'
In this example:
- /user/<username>: Defines a route that expects a username variable. Whatever value is provided in place of <username> will be passed as an argument to the profile() function;
- /post/<int:post_id>: Expects an integer post_id in the URL. The int converter ensures that the value captured is an integer;
- /path/<path:subpath>: Captures a path-like string (subpath) that can contain slashes. The path converter captures the entire string, including slashes, as a single argument.
When a user accesses these routes with varying inputs, Flask captures the specified variables and passes them as arguments to the corresponding view functions, allowing you to use these values within your logic to render different content, fetch specific data, or perform various operations based on the provided dynamic inputs.
What is the default method of route in Flask?
In Flask, the default method for a route is GET.
If you define a route using @app.route(‘/some_path’), it implicitly handles GET requests. This means that if you access that route via a web browser or through a simple link click, it’s treated as a GET request.
For example:
python
from flask import Flask app = Flask(__name__) @app.route('/hello') def hello(): return 'Hello, World!'
In this case, when you visit http://yourserver/hello in your browser, it’s a GET request that triggers the hello() function, and it responds with ‘Hello, World!’.
If you want a route to handle other HTTP methods (like POST, PUT, DELETE, etc.), you need to specify those methods explicitly using the methods parameter in the @app.route() decorator. For instance:
python
@app.route('/submit', methods=['POST']) def submit(): # Logic for handling form submissions return 'Form submitted successfully!'
This route /submit will only handle POST requests, and accessing it via a form submission using POST method would trigger this route’s functionality.
Conclusion
Flask’s routing capabilities extend far beyond the basic mapping of URLs to views. By mastering the intricacies of dynamic routes, variable rules, view responses, and leveraging Flask’s powerful tools like request objects and decorators, developers can craft robust applications.
This comprehensive understanding of routing equips developers to build scalable, adaptive, and sophisticated Flask applications that cater to dynamic user needs and evolving data structures. With this knowledge in hand, you’re poised to create exceptional, intelligent, and growth-oriented Flask applications.