Python descriptors are a less-talked-about, yet powerful feature in Python, often overshadowed by more popular elements. They provide a protocol for attribute access, allowing you to manage your class attributes in a more efficient and flexible way.
Understanding the Basics: What are Descriptors?
Descriptors in Python serve as a foundation for building more sophisticated and controlled interactions with class attributes. A descriptor is essentially a class that defines how a specific attribute should behave. It does this by implementing any of the special methods: __get__(), __set__(), or __delete__().
- __get__(self, instance, owner): Called to retrieve the attribute value;
- __set__(self, instance, value): Invoked to set the attribute value;
- __delete__(self, instance): Triggered when the attribute is deleted.
Each of these methods plays a unique role in managing how attributes of a class are accessed and modified, providing a high degree of control and customization.
The Magic Behind: How Descriptors Work
Descriptors shine by encapsulating attribute logic in a separate, reusable class. Consider a scenario where you need to ensure the values assigned to an attribute are always positive. Without descriptors, this would mean writing validation code wherever the attribute is set, leading to repetitive and error-prone code. With descriptors, this logic is centralized and abstracted away in a single class.
class PositiveValue:
def __set__(self, instance, value):
if value < 0:
raise ValueError("Value must be positive")
instance.__dict__[self.name] = value
def __get__(self, instance, owner):
return instance.__dict__.get(self.name, 0)
def __set_name__(self, owner, name):
self.name = name
Types of Descriptors: Data vs. Non-Data Descriptors
Descriptors in Python can be broadly categorized into two types:
- Data Descriptors: These include both __get__() and __set__() methods. They are typically used for managing data with validation, type-checking, or logging;
- Non-Data Descriptors: These only implement the __get__() method and are used primarily for methods and computed properties.
Descriptor Type | __get__() | __set__() | Use Case |
---|---|---|---|
Data Descriptor | Yes | Yes | Validation, Logging, Type-Checking |
Non-Data Descriptor | Yes | No | Methods, Computed Properties |
Implementing a Descriptor: A Step-by-Step Guide
Creating a descriptor involves a few straightforward steps:
- Define a descriptor class implementing any of the descriptor methods;
- Add an instance of this descriptor class as a class attribute to another class.
Here’s a simple example:
class MyClass:
positive_value = PositiveValue()
obj = MyClass()
obj.positive_value = 5 # Works fine
obj.positive_value = -5 # Raises ValueError
Real-World Applications: Enhancing Code with Descriptors
Descriptors are highly versatile and find use in various scenarios:
- Type Checking: Ensuring that attributes are of a specific type;
- Logging Attribute Access: Automatically logging whenever an attribute is accessed or changed;
- Lazy Computation: Deferring expensive computations until an attribute is accessed;
- Computed Properties: Dynamically calculating the value of an attribute.
Descriptors and Python’s Property Function
The property() function in Python is a built-in implementation of a data descriptor. It simplifies creating properties with optional getter, setter, and deleter methods. This is a more user-friendly way to create data descriptors for common use cases.
class MyClass:
def __init__(self, value):
self._value = value
@property
def value(self):
return self._value
@value.setter
def value(self, value):
if value < 0:
raise ValueError("Value must be positive")
self._value = value
Advanced Usage: Customizing Descriptors for Dynamic Behavior
In more sophisticated applications, Python’s descriptors can be tailored to exhibit dynamic behavior, adjusting their response based on varying circumstances. This feature is particularly useful in scenarios where the behavior of an attribute needs to adapt to different contexts or states. For instance, a descriptor could be engineered to provide different values for an attribute depending on which instance of a class is accessing it, or it might vary its behavior based on external conditions such as the time of day, the state of the application, or user-specific settings.
Consider a descriptor that returns data from a cache for some instances of a class but fetches fresh data for others. This level of dynamic response can make an application more efficient and adaptable. Such a descriptor would use the __get__ method to determine the context of the attribute access and then decide whether to return cached data or retrieve new information.
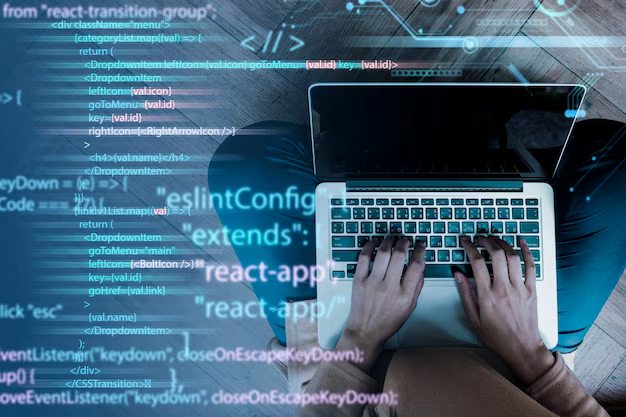
Descriptors and Python Internals: A Deep Dive
Delving into the realm of Python descriptors also provides an invaluable perspective on the internal workings of the Python language. By understanding descriptors, you gain insights into fundamental Python concepts such as method binding and how properties function under the hood.
Method binding, for example, is a process where functions defined in a class become methods of instances of that class. This is achieved through the use of non-data descriptors. The property function, widely used in Python, is an application of descriptors that allows for the creation of managed attributes, offering get, set, and delete functionalities in a clean, readable format. This deep dive into Python internals through descriptors enhances one’s ability to write more efficient and effective Python code and to understand why Python behaves the way it does in certain situations.
Common Pitfalls and Best Practices
Despite their power and flexibility, descriptors come with their own set of challenges and potential pitfalls. Their misuse can introduce unnecessary complexity into your code and can lead to subtle bugs that are difficult to trace and fix. Therefore, it is crucial to approach descriptors with a clear understanding of their behavior and to apply them judiciously.
One of the key best practices is to employ descriptors for scenarios that truly benefit from their unique capabilities, such as encapsulating complex attribute logic that is reused across different classes. On the other hand, for simpler tasks, where Python’s basic features suffice, it’s advisable to avoid the added complexity of descriptors.
Moreover, thorough testing becomes even more critical when working with descriptors. Due to their ability to alter the standard behavior of attribute access, it’s essential to ensure that they perform as expected in all scenarios and do not introduce unintended side effects. Regular and comprehensive testing will help ensure that descriptors add value to your code, enhancing its functionality without compromising its integrity.
Deep Copy in Python and its Interaction with Descriptors
Understanding deep copying in Python and its relationship with descriptors provides a comprehensive view of how Python manages object duplication, especially in the context of complex structures like those involving descriptors.
In Python, a deep copy creates a new compound object and then, recursively, inserts copies into it of the objects found in the original. This method is crucial when dealing with mutable objects or collections of objects, like lists or dictionaries, where simply copying the references (as in a shallow copy) would result in both the original and the copy pointing to the same nested objects.
import copy
original_list = [1, 2, [3, 4]]
deep_copied_list = copy.deepcopy(original_list)
When it comes to descriptors, the interaction with deep copying becomes nuanced. Descriptors, especially non-data descriptors that define methods, are typically shared between instances of a class, rather than being instance-specific. When you perform a deep copy of an object that includes descriptors, the copied object will still refer to the same descriptors as the original object. This behavior is generally desired and logical, as the descriptor’s methods should remain consistent across instances.
However, for data descriptors that maintain state (for example, caching computed values), a deep copy might be more complex. If the descriptor’s state is mutable and specific to an instance, then it’s crucial to ensure this state is also deep copied, to avoid shared mutable state between the original and the copy. This can be achieved by customizing the __deepcopy__ method in the descriptor class.
class StatefulDescriptor:
def __init__(self):
self.cache = {}
def __get__(self, instance, owner):
# Logic for fetching the value
return self.cache.get(instance)
def __set__(self, instance, value):
# Logic for setting the value
self.cache[instance] = value
def __deepcopy__(self, memo):
# Custom deep copy logic
copied_descriptor = StatefulDescriptor()
copied_descriptor.cache = copy.deepcopy(self.cache, memo)
return copied_descriptor
In this example, the StatefulDescriptor class includes a __deepcopy__ method to ensure that when an object containing this descriptor is deep copied, the descriptor’s cache is also deep copied, maintaining the isolation between the original and the copied object.
The relationship between deep copying and descriptors in Python highlights the intricacies of object copying and attribute management in Python, showcasing how deep understanding of these concepts can lead to more robust and reliable code.
Conclusion
Python descriptors are a potent tool, offering advanced attribute management that can elevate your code to a higher level of sophistication and efficiency. They allow for intricate control over how attributes are accessed and manipulated, promoting cleaner, more modular code. While powerful, they require careful use to maintain code readability and simplicity. Mastering descriptors not only enhances code functionality but also deepens understanding of Python’s core features, making them an essential skill for advanced Python programming.