Introduction
In the dynamic world of customer relationship management (CRM), Python emerges as a beacon of hope for businesses looking to enhance their customer interactions. This article dives deep into how to build a CRM with Python, particularly leveraging the power of Jupyter Notebooks.
Why Python for CRM?
Python is an excellent choice for Customer Relationship Management (CRM) development due to its simplicity, versatility, and robust libraries. This programming language offers a wide array of benefits that make it well-suited for creating CRM solutions. In this detailed discussion, we will explore these advantages, focusing on key aspects such as ease of use, rich libraries, and integration capabilities.
Key Benefits of Using Python for CRM:
Ease of Use
Python’s syntax is highly intuitive and easy to learn, making it accessible to both beginners and seasoned developers. This simplicity reduces the learning curve, allowing developers to quickly grasp the language and start building CRM applications efficiently.
Rich Libraries
Python boasts a vast ecosystem of libraries and packages that are indispensable for CRM development. Some of the most notable libraries include:
Library | Functionality |
---|---|
Pandas | Data manipulation and analysis |
NumPy | Numerical computations and array operations |
Matplotlib | Data visualization and charting |
Scikit-learn | Machine learning and predictive analytics |
SQLAlchemy | Database integration and query construction |
Requests | API interactions and data retrieval |
These libraries empower CRM developers to handle various data-related tasks efficiently, from processing customer data to generating insightful reports and visualizations.
Integration Capabilities
Python’s strength lies in its ability to seamlessly integrate with diverse databases and APIs. This feature is pivotal for CRM systems, as they often need to interact with external data sources, such as customer databases, third-party applications, or marketing platforms. Some integration options include:
- Database Integration: Python can connect to databases like MySQL, PostgreSQL, MongoDB, and others using libraries such as SQLAlchemy, enabling smooth data retrieval, storage, and manipulation;
- API Integration: Python’s ‘Requests’ library allows developers to communicate with APIs effectively, facilitating the exchange of data between the CRM system and external services;
- Web Scraping: Python’s libraries, such as BeautifulSoup and Scrapy, can be used for web scraping to gather valuable customer data from websites and online sources.
These integration capabilities enhance data accessibility and enable CRM systems to provide real-time, up-to-date information to users.
Setting Up Your Environment
To build a CRM with Python, start by setting up your environment. You’ll need Python installed on your machine along with Jupyter Notebooks, which offers an interactive coding environment.
Step 1: Install Python
Python is the programming language of choice for building many applications, including CRMs. Here’s how to get Python installed on your machine:
- Download Python: Visit the official Python website (https://www.python.org/downloads/) and download the latest version of Python that matches your operating system (Windows, macOS, or Linux);
- Installation: Run the downloaded installer and follow the on-screen instructions. Make sure to check the box that says “Add Python X.X to PATH” during installation, where “X.X” represents the Python version you are installing. This ensures that Python is accessible from the command line;
- Verify Installation: To confirm that Python is successfully installed, open your command line or terminal and type the following command: python –version;
- This should display the Python version you installed, indicating a successful installation.
Step 2: Install Jupyter Notebooks
Jupyter Notebooks provide an excellent interactive coding environment that is particularly beneficial for prototyping and development. Here’s how to install Jupyter Notebooks using pip, Python’s package manager:
- Open Command Line: Open your command line or terminal;
- Install Jupyter: Use the following command to install Jupyter Notebooks: pip install jupyter. This command will download and install Jupyter along with its dependencies;
- Launch Jupyter: Once the installation is complete, you can launch Jupyter Notebooks by running the following command in your command line or terminal: jupyter notebook. This command will start the Jupyter Notebook server and open a web browser window displaying the Jupyter interface.
Crafting Your CRM with Jupyter Notebooks
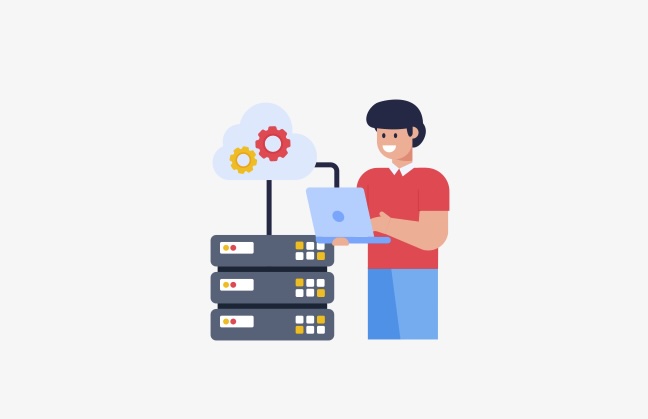
Jupyter Notebooks offers an interactive platform to write and test your Python code. Here, you can build a CRM with Python, testing each function as you go.
Data Management
Effective data management is essential for building a successful CRM system using Jupyter Notebooks. Python’s libraries, especially Pandas, play a pivotal role in this process. With Pandas, you can efficiently handle and manipulate customer data to meet your CRM needs.
- Import Pandas: Start by importing the Pandas library in your Jupyter Notebook with the following line of code: import pandas as pd;
- Create DataFrames: Utilize Pandas DataFrames to organize and store customer information, making it easy to manage and analyze;
- Data Transformation: Pandas offers various functions for data cleaning, transformation, and aggregation, ensuring your customer data is in the desired format;
- Data Integration: Seamlessly integrate data from different sources into your CRM system using Pandas, ensuring a unified and comprehensive view of your customers.
Task | Code Snippet |
---|---|
Import Pandas | import pandas as pd |
Create DataFrames | customer_data = pd.DataFrame({ … }) |
Data Transformation | Various Pandas functions |
Data Integration | Combining DataFrames, Merging Data |
Customer Interaction Tracking
Tracking customer interactions is a critical component of CRM, and Jupyter Notebooks, along with Python, provide an ideal environment for this task. In your CRM system, you can record interactions through various channels such as emails, calls, and meetings, and Python can be used to manage this data effectively.
- Record Keeping: Implement a structured approach to log customer interactions using Python classes or functions;
- Interaction Types: Capture details such as the type of interaction (e.g., email, call, meeting) and timestamp;
- Data Analysis: Utilize Python libraries like Pandas and Matplotlib to analyze interaction patterns, helping you gain valuable insights into customer behavior;
- Customization: Tailor your interaction tracking system to meet your specific CRM requirements and adapt it as your business evolves.
Task | Code Snippet |
---|---|
Record Keeping | Define classes/functions for interaction logging |
Interaction Types | Include interaction type and timestamp |
Data Analysis | Use Pandas for analysis, Matplotlib for visualization |
Customization | Adapt and extend the tracking system as needed |
Sales Forecasting
Sales forecasting is a vital aspect of CRM, enabling businesses to make informed decisions. Jupyter Notebooks, in combination with Python’s machine learning libraries, can facilitate this process. You can implement techniques like Regression Analysis and Time Series Analysis to predict future sales accurately.
- Regression Analysis: Use Python’s scikit-learn library to build regression models based on historical sales data;
- Time Series Analysis: Employ libraries like statsmodels to analyze trends and patterns over time, providing valuable insights for forecasting;
- Model Evaluation: Assess the accuracy and reliability of your sales forecasting models using appropriate metrics;
- Continuous Improvement: Continuously refine and update your sales forecasting models to adapt to changing market conditions.
Task | Code Snippet |
---|---|
Regression Analysis | from sklearn.linear_model import LinearRegression |
Time Series Analysis | import statsmodels.api as sm |
Model Evaluation | Utilize evaluation metrics like RMSE, MAE |
Continuous Improvement | Regularly update models based on new data |
Practical Examples
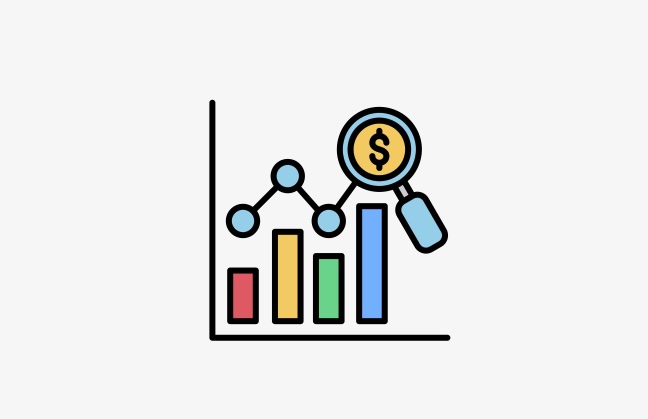
Let’s dive into some code examples to illustrate how to build a CRM with Python.
Example 1: Data Management
Data management is a fundamental aspect of any CRM system. In this example, we will show you how to load and manipulate customer data using the pandas library.
Code Example:
import pandas as pd
# Load customer data from a CSV file
customer_data = pd.read_csv('customers.csv')
# Display the first 5 rows of the customer data
print(customer_data.head())
In this code:
- We import the pandas library, a powerful tool for data manipulation in Python;
- We load customer data from a CSV file named ‘customers.csv’ using the pd.read_csv() function;
- We use print(customer_data.head()) to display the first 5 rows of the customer data.
This code snippet demonstrates how to read and inspect customer data, which is a crucial step in building a CRM system.
Example 2: Sales Forecasting
Sales forecasting is an essential feature of CRM systems. Here, we’ll use linear regression to predict future sales based on customer features. We’ll assume that you have already loaded and prepared the customer data as shown in Example 1.
Code Example:
from sklearn.linear_model import LinearRegression
# Prepare your data here (make sure 'customer_data' is loaded and preprocessed)
X = customer_data[['feature1', 'feature2']] # Features used for prediction
y = customer_data['sales'] # Target variable (sales)
# Create and train the linear regression model
model = LinearRegression().fit(X, y)
# Predict future sales based on new features
new_feature1 = 10 # Replace with the actual feature value
new_feature2 = 20 # Replace with the actual feature value
predicted_sales = model.predict([[new_feature1, new_feature2]])
# Print the predicted sales
print("Predicted Sales:", predicted_sales)
In this code:
- We import the LinearRegression class from the scikit-learn library, a popular library for machine learning in Python;
- We prepare the data by selecting relevant features (e.g., ‘feature1’ and ‘feature2’) and the target variable (‘sales’);
- We create an instance of the linear regression model and train it on the prepared data using LinearRegression().fit(X, y);
- We then use the trained model to predict future sales based on new feature values and display the result.
This example demonstrates how to implement sales forecasting within your CRM system using linear regression, a commonly used technique for predicting numerical values.
Conclusion
Building a CRM with Python using Jupyter Notebooks is an efficient and effective way to manage customer relationships. Its simplicity, combined with powerful libraries, makes Python a top choice for CRM development. Whether you’re a seasoned developer or a beginner, Python opens up a world of possibilities in the realm of customer relationship management.
FAQs
Absolutely! Python’s flexibility allows for easy integration with other systems through APIs
Yes, Python is scalable and can handle large datasets efficiently, especially with libraries like Pandas and NumPy.
Python itself is secure, but ensure best practices in coding and data storage to maintain high security.