Introduction
Programming with Python, a popular and flexible language, is easy and fun because to its many useful features. Two of these features, args and kwargs, are very useful tools that make Python functions more versatile and powerful. With a sprinkle of helpful examples and hints, this article will explore the meaning, usage, and development of args and kwargs, as well as how to create functions that can handle a variable number of arguments.
Python arguments: A Beginner’s Guide
One of Python’s most useful features, *args, lets you handle argument lists of variable length in functions. This comes in handy especially when you need to make functions that are adaptable and can take different amounts of arguments. This tutorial will go over the syntax, features, and usage of *args in great depth.
Syntax
The syntax for passing arguments as an array is simple. To do this, you must include the string *args as a parameter in the function definition. Here’s how it looks:
def my_function(*args): # Function implementation |
You can call the function with an unlimited number of positional parameters by using the *args parameter.
Functionality
In most cases, a function will make use of the *args parameter in order to accept an arbitrary number of arguments. The tuple that is created from the positional arguments of the function is compiled. After that, the function is able to handle this tuple correctly in accordance with the criteria.
def my_function(*args): for arg in args: print(arg) # Example usage my_function(1, 2, 3, 4) |
To illustrate how *args collects all specified values in a tuple, consider the following example: calling my_function(1, 2, 3, 4). This would output each argument.
Usage
Mastering the art of *args use is crucial. Internally, the function can index the arguments given through *args in the same way that it can access entries in a list. In order to demonstrate its use, let’s examine a few examples:
Description | Function Name | Function Code | Usage Example | Output |
---|---|---|---|---|
Sum of Arguments | calculate_sum | def calculate_sum(*args):\n total = 0\n for arg in args:\n total += arg\n return total | result = calculate_sum(1, 2, 3, 4, 5)\nprint(“Sum:”, result) | “Sum: 15” |
Concatenating Strings | concatenate_strings | def concatenate_strings(*args):\n result = “”\n for arg in args:\n result += arg\n return result | final_string = concatenate_strings(“Hello, “, “World!”, ” How are you?”)\nprint(“Concatenated String:”, final_string) | “Concatenated String: Hello, World! How are you?” |
Finding the Maximum Value | find_max | def find_max(*args):\n if not args:\n return None\n max_val = args[0]\n for arg in args:\n if arg > max_val:\n max_val = arg\n return max_val | max_value = find_max(42, 17, 94, 23, 61)\nprint(“Maximum Value:”, max_value) | “Maximum Value: 94” |
In this example, we find the maximum value from a variable number of arguments passed to the function.
Why Use args?
Flexibility
Flexibility is one of the main reasons why *args are used in Python calls. When you use *args, functions can take any number of inputs. Instead, they can take any number of positional inputs, which means they can be used in a variety of situations. You can use this freedom to make functions that can handle a wide range of inputs.
Convenience
Another significant advantage of *args is its convenience, especially when you need to iterate through multiple arguments within a function. Without *args, you would have to define a function with a specific number of parameters, which can be cumbersome and impractical when you don’t know the exact number of arguments in advance. With *args, you can loop through the arguments effortlessly, simplifying your code.
Practical Example
Let’s delve into a practical example to better understand the use of *args in Python functions.
def add_numbers(*args): return sum(args) result = add_numbers(1, 2, 3, 4) print(result) # Output: 10 |
In this example, we have defined a function called add_numbers that takes advantage of the *args notation. The function accepts any number of arguments and calculates their sum using the sum() function.
Here’s a breakdown of how this code works:
- We define the add_numbers function and specify *args as its parameter. This allows the function to accept an arbitrary number of arguments;
- Inside the function, we use the sum() function to calculate the sum of all the arguments passed to it. This is achieved by passing the args parameter to sum(), which automatically handles the addition of all the values;
- We call the add_numbers function with four arguments: 1, 2, 3, and 4;
- Finally, we print the result, which is the sum of the arguments, in this case, 10.
Exploring kwargs in Python
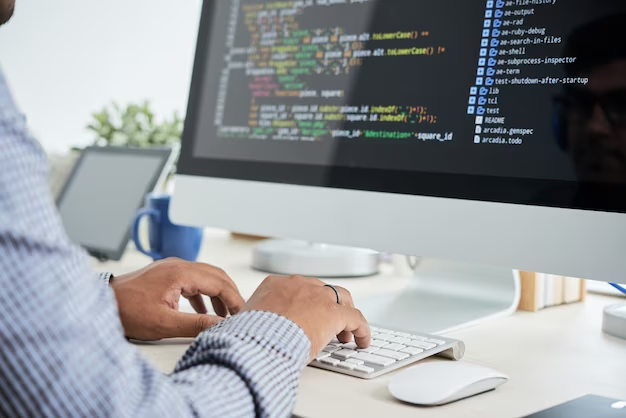
kwargs is a special notation in Python that enables a function to accept an arbitrary number of keyword arguments. These keyword arguments are passed to the function as a dictionary, allowing you to work with variable-length key-value pairs effortlessly.
Syntax
In Python, you can use kwargs in a function definition by prefixing the parameter name with double asterisks () as follows:
def example_function(**kwargs): # Function body |
The **kwargs notation indicates that the function can accept keyword arguments in the form of a dictionary.
Functionality
The primary functionality of **kwargs is to allow the passing of variable-length key-value pairs to a function. When you use **kwargs in a function, it collects all the keyword arguments provided during the function call and stores them in a dictionary. Each keyword argument becomes a key in the dictionary, and its associated value becomes the corresponding value in the dictionary. Here’s how **kwargs works in practice:
def example_function(**kwargs): for key, value in kwargs.items(): print(f”{key}: {value}”) # Calling the function with keyword arguments example_function(name=”Alice”, age=30, city=”New York”) |
Output:
name: Alice age: 30 city: New York |
In this example, the example_function accepts keyword arguments (name, age, and city) and iterates through them, printing each key-value pair.
Usage
**kwargs is particularly useful in situations where you want to create functions that are adaptable to different sets of keyword arguments. Some common use cases for **kwargs include:
- Configuring functions with various options;
- Passing additional parameters to other functions;
- Creating flexible and extensible APIs.
Configuring a Function Example:
def configure_settings(**kwargs): settings = { “color”: “red”, “size”: “medium”, “border”: “dotted”, } settings.update(kwargs) print(“Updated settings:”, settings) # Configuring settings using **kwargs configure_settings(color=”blue”, size=”large”, background=”white”) |
Output:
Updated settings: {‘color’: ‘blue’, ‘size’: ‘large’, ‘border’: ‘dotted’, ‘background’: ‘white’} |
In this example, the configure_settings function has default settings but can be customized using **kwargs.
Why Use kwargs?
Readability
One of the primary reasons for using **kwargs in Python functions is to enhance code readability. By allowing functions to accept named arguments, **kwargs improves the clarity of your code. Named arguments make it explicit and easy to understand the purpose of each parameter when calling a function, which can be particularly valuable in collaborative or large codebases.
Flexibility
**kwargs offers a significant advantage in terms of flexibility. It enables you to add new keyword arguments to a function without necessitating alterations to existing function calls. This flexibility is especially useful when you want to extend the functionality of a function without disrupting the code that relies on it. It ensures that your codebase remains maintainable and adaptable over time.
Syntax
In Python, you can employ kwargs in a function definition by prefixing the parameter name with double asterisks () as follows:
def example_function(**kwargs): # Function body |
The **kwargs notation indicates that the function can accept keyword arguments in the form of a dictionary.
Practical Example
To better grasp the significance of **kwargs, let’s examine a practical example:
def print_pet_names(**kwargs): for pet, name in kwargs.items(): print(f”{pet}: {name}”) # Calling the function with keyword arguments print_pet_names(dog=”Rover”, cat=”Whiskers”) |
Output:
makefile Copy code dog: Rover cat: Whiskers |
In this example, we have defined a function called print_pet_names that utilizes **kwargs. The function accepts keyword arguments, where the pet’s name is associated with its respective name.
How the Code Works
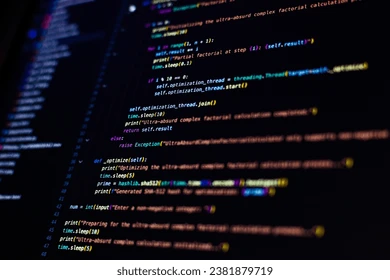
Here’s a breakdown of how this code works:
- We define the print_pet_names function with the parameter **kwargs, indicating that it can accept any number of keyword arguments;
- Inside the function, we use a for loop to iterate through the dictionary of keyword arguments provided via **kwargs. For each key-value pair, we print the pet’s name and its associated name;
- We call the print_pet_names function with two keyword arguments: dog=”Rover” and cat=”Whiskers”. As a result, the function prints the names of the two pets.
Developing Functions with Variable Number of Arguments
Python allows you to create highly flexible functions that can accept a variable number of arguments by using the *args and **kwargs features. This guide will walk you through the process of developing such functions step by step.
Define the Function
To create a function that can accept a variable number of arguments, you should define it with both *args and **kwargs in the function signature. Here’s an example of how to define such a function:
def create_profile(name, email, *args, **kwargs): # Function implementation goes here |
In the function definition above, name and email are regular positional arguments, while *args and **kwargs allow us to accept a variable number of additional arguments.
Process args
If you need to access each of the positional arguments passed to the function, you can iterate over the *args tuple. The *args variable collects all the additional positional arguments passed to the function as a tuple. Here’s an example of how to process *args:
def create_profile(name, email, *args, **kwargs): profile = {‘name’: name, ’email’: email, ‘interests’: args, ‘other_info’: kwargs} # Additional processing of args can be done here return profile |
In the example above, we’ve included the args as part of the profile dictionary to store the additional positional arguments.
Handle kwargs
The **kwargs variable allows you to accept named arguments as a dictionary. You can access these named arguments just like you would with any dictionary. Here’s how to handle **kwargs:
def create_profile(name, email, *args, **kwargs): profile = {‘name’: name, ’email’: email, ‘interests’: args, ‘other_info’: kwargs} # Additional processing of kwargs can be done here return profile |
In the example above, we’ve included the kwargs as part of the profile dictionary to store the additional named arguments.
Example
Let’s put it all together with an example:
def create_profile(name, email, *args, **kwargs): profile = {‘name’: name, ’email’: email, ‘interests’: args, ‘other_info’: kwargs} # Additional processing of args and kwargs can be done here return profile user_profile = create_profile(‘John Doe’, ‘[email protected]’, ‘reading’, ‘gaming’, age=30, country=’USA’) |
In this example, create_profile is called with the name and email as positional arguments and additional interests, age, and country as both positional and named arguments. The function returns a dictionary containing all the information.
When to Use args and kwargs
Alt: args and kwargs
In Python, the use of *args and **kwargs allows developers to create functions with variable numbers of arguments. These features offer flexibility and adaptability in handling different argument scenarios. Let’s explore when and how to use *args and **kwargs effectively, along with examples.
Using *args
*args is used when the number of positional arguments is unknown or can vary. It collects any additional positional arguments passed to a function into a tuple, allowing the function to work with them dynamically. Common use cases for *args include:
- Handling a variable number of arguments;
- When you want to create a function that can accept any number of arguments without explicitly defining each one.
Example of using *args:
def calculate_sum(*args): result = 0 for num in args: result += num return result sum_result = calculate_sum(1, 2, 3, 4, 5) |
In this example, calculate_sum can accept any number of arguments, and it dynamically calculates their sum.
Using **kwargs
**kwargs is used when you need to handle named arguments dynamically. It collects additional named arguments passed to a function into a dictionary, allowing you to access and process them with ease. Common use cases for **kwargs include:
- When you want to pass a varying number of named arguments;
- Creating functions with optional parameters that can be customized by users.
Example of using **kwargs:
def create_person(**kwargs): person = {} for key, value in kwargs.items(): person[key] = value return person person_info = create_person(name=’John’, age=30, city=’New York’) |
In this example, create_person accepts a dynamic set of named arguments, creating a dictionary containing the person’s information.
Combining *args and **kwargs
You can use both *args and **kwargs in a function definition to achieve maximum flexibility. This combination allows you to handle both positional and named arguments dynamically, making your functions extremely versatile.
Example of combining *args and **kwargs:
def process_data(title, *args, **kwargs): result = { ‘title’: title, ‘args’: args, ‘kwargs’: kwargs } return result data = process_data(‘Sample Data’, 1, 2, 3, param1=’value1′, param2=’value2′) |
In this example, process_data accepts a title as a positional argument, followed by any number of positional arguments (*args) and named arguments (**kwargs).
Conclusion
args and kwargs in Python are invaluable tools for creating flexible, dynamic functions. By understanding and utilizing these features, programmers can write more adaptable and efficient code. Remember, with great power comes great responsibility: use args and kwargs wisely to maintain code clarity and readability.
FAQs
Absolutely! They can be combined in a single function to handle both positional and keyword arguments.
It will result in an error. *args is only for non-keyword arguments.
Yes, they can coexist with default arguments in a function.
While they add flexibility, overusing them can make the code less readable and harder to debug.
Just like in standalone functions, they allow methods to accept a variable number of arguments.